生成只用encoder部分建立模型然后线性输出,实现多输入单输出的keras框架下的时间序列预测模型
时间: 2024-02-06 13:10:27 浏览: 31
在Keras框架下,你可以使用Functional API来实现多输入单输出的时间序列预测模型。具体步骤如下:
1. 导入所需的库和模块
```python
from keras.layers import Input, Dense, concatenate
from keras.models import Model
```
2. 定义输入层
假设我们有两个时间序列作为输入,分别为X1和X2,它们的形状分别为(n_samples, n_timesteps1, n_features1)和(n_samples, n_timesteps2, n_features2),其中n_samples表示样本数量,n_timesteps1和n_timesteps2表示每个时间序列的时间步数,n_features1和n_features2表示每个时间步的特征数。我们可以使用Input函数来定义输入层:
```python
input1 = Input(shape=(n_timesteps1, n_features1))
input2 = Input(shape=(n_timesteps2, n_features2))
```
3. 定义编码器层
我们可以使用Dense层来定义编码器层,将每个时间序列压缩成一个固定长度的向量。假设我们的编码器层的大小为encoding_dim,我们可以这样定义:
```python
encoder1 = Dense(encoding_dim, activation='relu')(input1)
encoder2 = Dense(encoding_dim, activation='relu')(input2)
```
4. 合并编码器层
我们可以使用concatenate函数将两个编码器层合并为一个。
```python
merged = concatenate([encoder1, encoder2])
```
5. 定义输出层
我们可以使用Dense层来定义输出层,将合并后的编码器层转换为我们需要的输出形状。假设我们希望输出一个形状为(n_samples, n_predictions, n_features)的时间序列,其中n_predictions表示未来的时间步数,我们可以这样定义:
```python
output = Dense(n_predictions * n_features, activation='linear')(merged)
output = Reshape((n_predictions, n_features))(output)
```
6. 定义模型
我们可以使用Model函数定义模型,指定输入和输出层:
```python
model = Model(inputs=[input1, input2], outputs=output)
```
7. 编译模型
我们可以使用compile函数编译模型,指定损失函数和优化器:
```python
model.compile(loss='mse', optimizer='adam')
```
8. 训练模型
我们可以使用fit函数训练模型,传入两个时间序列作为输入和一个时间序列作为输出:
```python
model.fit([X1, X2], y, epochs=epochs, batch_size=batch_size)
```
其中X1和X2分别为两个时间序列的输入数据,y为输出数据,epochs表示训练的轮数,batch_size表示每批次训练的样本数量。
完整代码如下:
```python
from keras.layers import Input, Dense, concatenate, Reshape
from keras.models import Model
# 定义输入层
input1 = Input(shape=(n_timesteps1, n_features1))
input2 = Input(shape=(n_timesteps2, n_features2))
# 定义编码器层
encoder1 = Dense(encoding_dim, activation='relu')(input1)
encoder2 = Dense(encoding_dim, activation='relu')(input2)
# 合并编码器层
merged = concatenate([encoder1, encoder2])
# 定义输出层
output = Dense(n_predictions * n_features, activation='linear')(merged)
output = Reshape((n_predictions, n_features))(output)
# 定义模型
model = Model(inputs=[input1, input2], outputs=output)
# 编译模型
model.compile(loss='mse', optimizer='adam')
# 训练模型
model.fit([X1, X2], y, epochs=epochs, batch_size=batch_size)
```
相关推荐
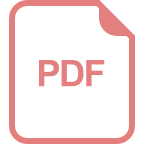
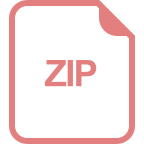
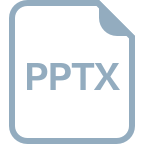














