Android开发中使用Java解析json数据并使用
时间: 2024-05-16 13:19:31 浏览: 156
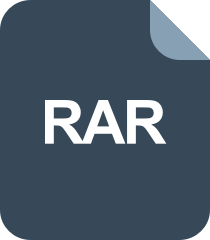
android 解析json数据

在Android开发中,可以使用Java解析JSON数据,并将解析后的数据用于应用程序中。
以下是一种常见的解析JSON数据的方法:
1. 获取JSON数据
在Android应用程序中,可以使用HttpURLConnection或OkHttp等库来获取JSON数据。以下是使用HttpURLConnection获取JSON数据的示例代码:
```java
URL url = new URL("http://example.com/data.json");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.connect();
InputStream inputStream = connection.getInputStream();
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream));
String line;
StringBuilder stringBuilder = new StringBuilder();
while ((line = bufferedReader.readLine()) != null) {
stringBuilder.append(line);
}
String jsonString = stringBuilder.toString();
```
2. 解析JSON数据
可以使用Java标准库中的JSONObject和JSONArray类来解析JSON数据。以下是一个简单的解析JSON数据的示例代码:
```java
JSONObject jsonObject = new JSONObject(jsonString);
JSONArray jsonArray = jsonObject.getJSONArray("data");
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject item = jsonArray.getJSONObject(i);
String name = item.getString("name");
int age = item.getInt("age");
// ...
}
```
上述代码首先将JSON字符串转换为JSONObject,然后从中获取名为"data"的JSONArray,遍历这个数组,从每个JSONObject中获取需要的数据。
3. 使用解析后的数据
可以将解析后的数据用于应用程序中,例如显示在UI控件中。以下是一个简单的示例代码:
```java
TextView textView = findViewById(R.id.text_view);
textView.setText(name + ", " + age);
```
这是一个简单的Android应用程序中使用Java解析JSON数据的示例。在实际应用中,可能需要根据具体需求进行更复杂的JSON数据解析和数据处理。
阅读全文
相关推荐
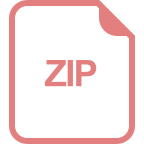
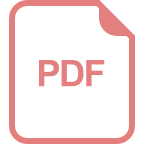
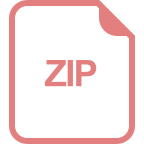
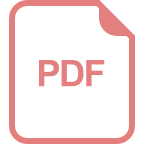
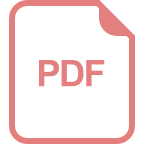
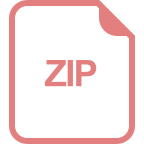
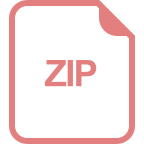
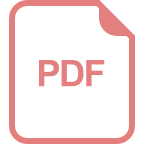
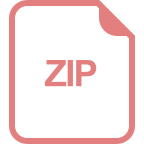
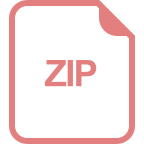
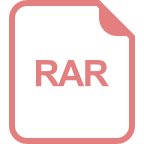
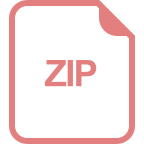
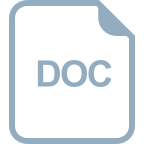
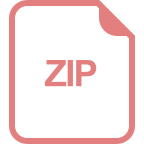
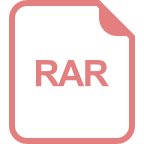
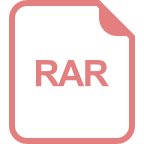