编写程序,用数组分别建两个堆栈共享,输入一序列整数,将奇数和偶数分别存放在两个栈中,栈满后打印
时间: 2024-05-15 19:13:10 浏览: 143
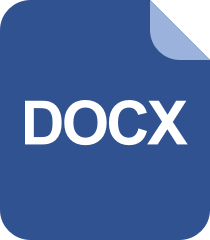
两个栈空间共享,栈满打印
下面是使用C++语言编写的程序:
```cpp
#include <iostream>
#define MAXSIZE 10
using namespace std;
class Stack {
private:
int top1, top2;
int data[MAXSIZE];
public:
Stack() {
top1 = -1;
top2 = MAXSIZE;
}
bool isFull() {
return top1 + 1 == top2;
}
bool isEmpty(int stackNum) {
if (stackNum == 1) {
return top1 == -1;
} else {
return top2 == MAXSIZE;
}
}
void push(int stackNum, int value) {
if (isFull()) {
cout << "Stack is full!" << endl;
return;
}
if (stackNum == 1) {
top1++;
data[top1] = value;
} else {
top2--;
data[top2] = value;
}
}
int pop(int stackNum) {
if (isEmpty(stackNum)) {
cout << "Stack is empty!" << endl;
return -1;
}
if (stackNum == 1) {
int value = data[top1];
top1--;
return value;
} else {
int value = data[top2];
top2++;
return value;
}
}
};
int main() {
Stack s;
int n, x;
cout << "Enter the number of integers: ";
cin >> n;
cout << "Enter the integers:" << endl;
for (int i = 0; i < n; i++) {
cin >> x;
if (x % 2 == 0) {
s.push(1, x);
} else {
s.push(2, x);
}
}
cout << "Even numbers: ";
while (!s.isEmpty(1)) {
cout << s.pop(1) << " ";
}
cout << endl;
cout << "Odd numbers: ";
while (!s.isEmpty(2)) {
cout << s.pop(2) << " ";
}
cout << endl;
return 0;
}
```
程序中使用了一个名为 `Stack` 的类来表示共享堆栈,它包含两个栈指针 `top1` 和 `top2`,表示两个栈的栈顶元素位置,以及一个数组 `data`,用来存储栈中的元素。
类中提供了一些方法,比如 `isFull` 用来判断堆栈是否满了,`isEmpty` 用来判断某个栈是否为空,`push` 用来将元素压入堆栈,`pop` 用来从堆栈中弹出元素。
在主函数中,先输入整数序列,并将奇数和偶数分别压入两个栈中,最后依次输出两个栈中的元素。
阅读全文
相关推荐
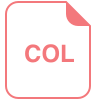
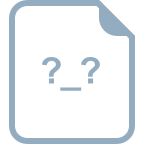
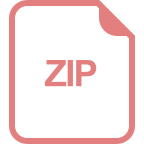
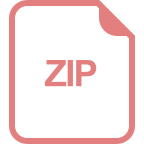
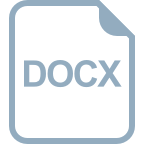
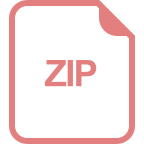
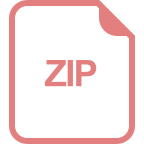