string find函数
时间: 2023-10-11 16:13:29 浏览: 72
`find` 函数是字符串类中的一个成员函数,用于在一个字符串中查找一个子字符串。它的用法如下:
```c++
size_t find(const string& str, size_t pos = 0) const;
```
其中,`str` 表示要查找的子字符串,`pos` 表示开始查找的位置,默认值为 `0`。`find` 函数返回查找到的子字符串在原字符串中的位置,如果没有找到则返回 `string::npos`。
例如,如果要在字符串 `s` 中查找子字符串 `"hello"`,可以使用以下代码:
```c++
string s = "world hello world";
size_t pos = s.find("hello");
if (pos != string::npos) {
cout << "Found at position " << pos << endl;
} else {
cout << "Not found" << endl;
}
```
输出结果为:
```
Found at position 6
```
表示子字符串 `"hello"` 在原字符串中的位置为 6。
相关问题
string find函数参数
find函数的参数是两个字符串:被搜索的字符串和要查找的子字符串。具体格式如下:
```
int find(const string& str, const string& sub_str);
```
其中,`str`是被搜索的字符串,`sub_str`是要查找的子字符串。该函数会返回子字符串在被搜索的字符串中第一次出现的位置。如果找不到子字符串,会返回一个特殊值`string::npos`。
python string find函数
Python中的字符串有一个内置的`find()`函数,用于查找子字符串在字符串中的位置。
`find()`函数的语法如下:
```python
str.find(sub, start, end)
```
- `sub`:要查找的子字符串。
- `start`(可选):搜索的起始位置,默认为0。
- `end`(可选):搜索的结束位置,默认为字符串的长度。
`find()`函数返回子字符串在字符串中第一次出现的索引,如果找不到则返回-1。
下面是一个示例:
```python
sentence = "I love Python programming"
index = sentence.find("Python")
print(index) # 输出:7
```
在上面的例子中,子字符串"Python"在原字符串中的索引位置是7。
阅读全文
相关推荐
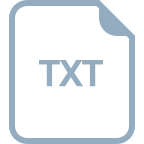
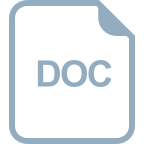












