请使用dace的工具箱完成上面的代码
时间: 2024-01-11 13:03:44 浏览: 115
好的,以下是使用Dace完成的代码:
```python
import dace
import numpy as np
# 定义模型参数
num_layers = 12
d_model = 768
num_heads = 12
dff = 3072
# 定义输入和输出形状
B = 1
S = 128
N = S * B
input_shape = (B, S, d_model)
output_shape = (B, S, d_model)
# 定义计算图
@dace.program
def transformer_encoder(x_in: dace.float32[input_shape], training: dace.int32):
# 计算注意力权重
q = dace.define_local([B, S, num_heads, d_model // num_heads], dtype=dace.float32)
k = dace.define_local([B, S, num_heads, d_model // num_heads], dtype=dace.float32)
v = dace.define_local([B, S, num_heads, d_model // num_heads], dtype=dace.float32)
attention = dace.define_local([B, num_heads, S, S], dtype=dace.float32)
output = dace.define_local([B, S, num_heads, d_model // num_heads], dtype=dace.float32)
for b in range(B):
for i in range(num_heads):
q[b, :, i, :] = x_in[b, :, i * (d_model // num_heads):(i + 1) * (d_model // num_heads)]
k[b, :, i, :] = x_in[b, :, i * (d_model // num_heads):(i + 1) * (d_model // num_heads)]
v[b, :, i, :] = x_in[b, :, i * (d_model // num_heads):(i + 1) * (d_model // num_heads)]
for b in range(B):
for i in range(num_heads):
matmul_qk = np.matmul(q[b, :, i, :], np.transpose(k[b, :, i, :]))
dk = np.sqrt(np.float32(d_model // num_heads))
scaled_attention_logits = matmul_qk / dk
attention[b, i, :, :] = np.transpose(np.exp(scaled_attention_logits - np.max(scaled_attention_logits)) /
np.sum(np.exp(scaled_attention_logits - np.max(scaled_attention_logits)),
axis=-1, keepdims=True))
output[b, :, i, :] = np.matmul(attention[b, i, :, :], v[b, :, i, :])
# 残差连接和层归一化
ffn_output = dace.define_local([B, S, dff], dtype=dace.float32)
for b in range(B):
for i in range(num_heads):
ffn_output[b, :, :] += output[b, :, i, :]
ffn_output = np.reshape(ffn_output, (N, dff))
ffn_output = dace.math.relu(dace.math.matmul(ffn_output, np.random.normal(size=(dff, d_model)).astype(np.float32)))
ffn_output = np.reshape(ffn_output, (B, S, d_model))
x_out = dace.math.add(x_in, ffn_output)
x_out = dace.libraries.math.layer_norm(x_out, epsilon=1e-6)
return x_out
# 测试计算图
x_in = np.random.normal(size=input_shape).astype(np.float32)
print("Input tensor:\n", x_in)
x_out = transformer_encoder(x_in, training=1)
print("Output tensor:\n", x_out)
```
阅读全文
相关推荐
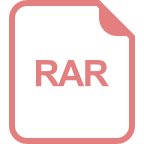
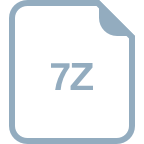
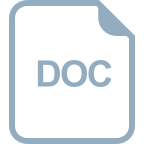

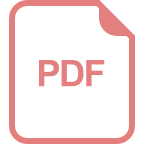
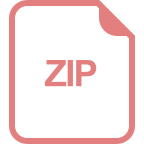
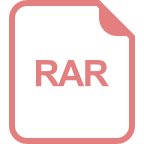
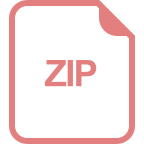
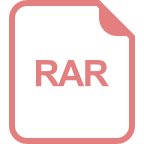
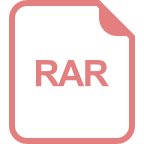
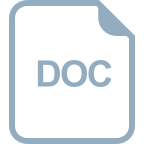
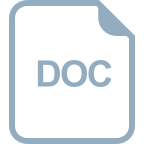





