c语言西电1.图书馆管理系统
时间: 2024-02-15 20:37:12 浏览: 30
这是一个比较大的项目,需要设计数据库、界面和逻辑等多个方面。以下是一个简单的图书馆管理系统的示例代码,供参考:
```c
#include <stdio.h>
#include <string.h>
// 图书信息结构体
typedef struct {
char id[10]; // 图书编号
char name[50]; // 图书名称
char author[50]; // 作者
int count; // 数量
} Book;
// 学生信息结构体
typedef struct {
char id[10]; // 学号
char name[50]; // 姓名
int borrow_count; // 借阅数量
Book books[5]; // 借阅的图书
} Student;
// 学生列表
Student students[100];
int student_num = 0;
// 图书列表
Book books[100];
int book_num = 0;
// 初始化图书列表
void init_books() {
books[0] = (Book){"001", "C语言程序设计", "谭浩强", 10};
books[1] = (Book){"002", "Java编程思想", "Bruce Eckel", 5};
book_num = 2;
}
// 初始化学生列表
void init_students() {
student_num = 0;
}
// 根据图书编号查找图书
Book* find_book(char* id) {
for (int i = 0; i < book_num; i++) {
if (strcmp(books[i].id, id) == 0) {
return &books[i];
}
}
return NULL;
}
// 根据学生学号查找学生
Student* find_student(char* id) {
for (int i = 0; i < student_num; i++) {
if (strcmp(students[i].id, id) == 0) {
return &students[i];
}
}
return NULL;
}
// 借书
void borrow_book(char* student_id, char* book_id) {
Student* student = find_student(student_id);
if (student == NULL) {
printf("学生不存在!\n");
return;
}
Book* book = find_book(book_id);
if (book == NULL) {
printf("图书不存在!\n");
return;
}
if (book->count <= 0) {
printf("图书已被借完!\n");
return;
}
if (student->borrow_count >= 5) {
printf("借阅数量已达上限!\n");
return;
}
student->books[student->borrow_count] = *book;
student->borrow_count++;
book->count--;
printf("借书成功!\n");
}
// 还书
void return_book(char* student_id, char* book_id) {
Student* student = find_student(student_id);
if (student == NULL) {
printf("学生不存在!\n");
return;
}
Book* book = find_book(book_id);
if (book == NULL) {
printf("图书不存在!\n");
return;
}
int index = -1;
for (int i = 0; i < student->borrow_count; i++) {
if (strcmp(student->books[i].id, book_id) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("学生未借阅该图书!\n");
return;
}
for (int i = index; i < student->borrow_count - 1; i++) {
student->books[i] = student->books[i + 1];
}
student->borrow_count--;
book->count++;
printf("还书成功!\n");
}
// 显示学生信息
void show_student(char* student_id) {
Student* student = find_student(student_id);
if (student == NULL) {
printf("学生不存在!\n");
return;
}
printf("学号:%s\n", student->id);
printf("姓名:%s\n", student->name);
printf("借阅数量:%d\n", student->borrow_count);
printf("借阅的图书:\n");
for (int i = 0; i < student->borrow_count; i++) {
printf(" %s 《%s》 %s\n", student->books[i].id, student->books[i].name, student->books[i].author);
}
}
// 显示图书信息
void show_book(char* book_id) {
Book* book = find_book(book_id);
if (book == NULL) {
printf("图书不存在!\n");
return;
}
printf("编号:%s\n", book->id);
printf("名称:%s\n", book->name);
printf("作者:%s\n", book->author);
printf("数量:%d\n", book->count);
}
// 显示所有图书
void show_all_books() {
printf("所有图书:\n");
for (int i = 0; i < book_num; i++) {
printf(" %s 《%s》 %s 数量:%d\n", books[i].id, books[i].name, books[i].author, books[i].count);
}
}
// 显示所有学生
void show_all_students() {
printf("所有学生:\n");
for (int i = 0; i < student_num; i++) {
printf(" %s %s 借阅数量:%d\n", students[i].id, students[i].name, students[i].borrow_count);
}
}
int main() {
init_books();
init_students();
while (1) {
printf("\n请选择操作:\n");
printf("1. 借书\n");
printf("2. 还书\n");
printf("3. 显示学生信息\n");
printf("4. 显示图书信息\n");
printf("5. 显示所有图书\n");
printf("6. 显示所有学生\n");
printf("7. 退出\n");
int choice = 0;
scanf("%d", &choice);
if (choice == 1) {
printf("请输入学生学号和图书编号:\n");
char student_id[10], book_id[10];
scanf("%s %s", student_id, book_id);
borrow_book(student_id, book_id);
} else if (choice == 2) {
printf("请输入学生学号和图书编号:\n");
char student_id[10], book_id[10];
scanf("%s %s", student_id, book_id);
return_book(student_id, book_id);
} else if (choice == 3) {
printf("请输入学生学号:\n");
char student_id[10];
scanf("%s", student_id);
show_student(student_id);
} else if (choice == 4) {
printf("请输入图书编号:\n");
char book_id[10];
scanf("%s", book_id);
show_book(book_id);
} else if (choice == 5) {
show_all_books();
} else if (choice == 6) {
show_all_students();
} else if (choice == 7) {
break;
} else {
printf("无效的操作!\n");
}
}
return 0;
}
```
该示例代码包含了借书、还书、显示学生信息、显示图书信息、显示所有图书和显示所有学生等功能。需要注意的是,该代码仅为示例,实际的图书馆管理系统需要根据具体需求进行设计和开发。
相关推荐
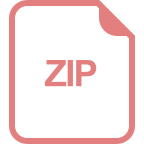
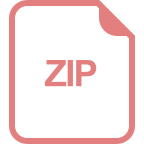















