java后端用线程池构建2个线程,线程1只取号码末尾数 in ('0','1','2','3','4'),线程2只取号码末尾数 in ('5','6','7','8','9');线程每次只取100条数据,遍历发送,100条遍历后休息5秒再次遍历,也就是while死循环,
时间: 2023-08-06 11:14:33 浏览: 78
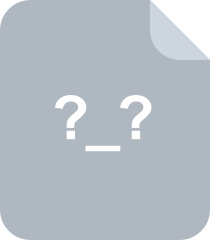
java中while循环.md
可以使用Java中的线程池ThreadPoolExecutor来实现:
```
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
public class NumberThread {
private static final int THREAD_POOL_SIZE = 2; // 线程池大小
private static final int BATCH_SIZE = 100; // 每次取的数据条数
private static final int SLEEP_TIME = 5000; // 休息时间,单位:毫秒
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(THREAD_POOL_SIZE);
// 线程1只取号码末尾数 in ('0','1','2','3','4')
executor.submit(() -> {
while (true) {
// 取数据
String[] numbers = getData("0", "1", "2", "3", "4", BATCH_SIZE);
// 遍历发送
for (String number : numbers) {
send(number);
}
// 休息
try {
TimeUnit.MILLISECONDS.sleep(SLEEP_TIME);
} catch (InterruptedException e) {
// ignore
}
}
});
// 线程2只取号码末尾数 in ('5','6','7','8','9')
executor.submit(() -> {
while (true) {
// 取数据
String[] numbers = getData("5", "6", "7", "8", "9", BATCH_SIZE);
// 遍历发送
for (String number : numbers) {
send(number);
}
// 休息
try {
TimeUnit.MILLISECONDS.sleep(SLEEP_TIME);
} catch (InterruptedException e) {
// ignore
}
}
});
}
private static String[] getData(String... ends) {
// TODO: 根据末尾数取数据,返回字符串数组
}
private static void send(String number) {
// TODO: 发送数据
}
}
```
其中,getData方法中可以使用JDBC访问数据库,根据SQL查询语句取出符合条件的数据,然后返回字符串数组;send方法中可以使用HttpClient或者其他HTTP客户端发送数据。
阅读全文
相关推荐
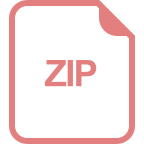

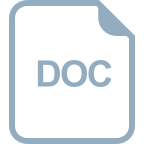
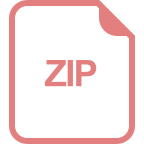
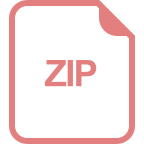
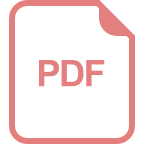
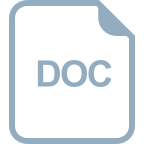
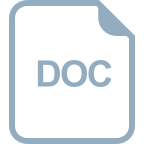
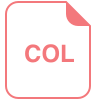
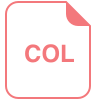
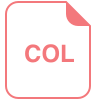
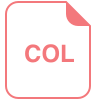
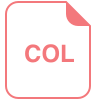
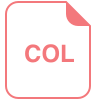
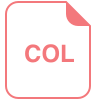
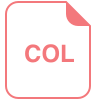
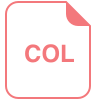