用c语言写一个贪吃蛇游戏
时间: 2024-05-07 15:19:19 浏览: 7
以下是用 C 语言实现的简单贪吃蛇游戏代码,供参考:
```c
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <windows.h>
#define WIDTH 40 // 游戏区域宽度
#define HEIGHT 20 // 游戏区域高度
#define MAXLEN (WIDTH * HEIGHT) // 蛇的最大长度
// 定义方向常量
#define UP 0
#define RIGHT 1
#define DOWN 2
#define LEFT 3
// 定义蛇的结构体
struct Snake {
int x[MAXLEN], y[MAXLEN]; // 蛇身上所有节点的坐标
int len; // 蛇的长度
int dir; // 蛇的方向
};
// 定义食物的结构体
struct Food {
int x, y; // 食物的坐标
};
// 定义游戏区域的二维数组
char game[HEIGHT][WIDTH];
// 初始化游戏区域
void init_game() {
int i, j;
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
game[i][j] = ' ';
}
}
}
// 画出游戏区域
void draw_game() {
int i, j;
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
if (i == 0 || i == HEIGHT - 1 || j == 0 || j == WIDTH - 1) {
printf("#");
} else {
printf("%c", game[i][j]);
}
}
printf("\n");
}
}
// 生成食物
void generate_food(struct Food *food) {
food->x = rand() % (WIDTH - 2) + 1;
food->y = rand() % (HEIGHT - 2) + 1;
game[food->y][food->x] = '@';
}
// 初始化蛇
void init_snake(struct Snake *s) {
s->x[0] = WIDTH / 2;
s->y[0] = HEIGHT / 2;
s->len = 1;
s->dir = RIGHT;
game[s->y[0]][s->x[0]] = 'O';
}
// 移动蛇
void move_snake(struct Snake *s, struct Food *food, int *score) {
int i;
int tail_x = s->x[s->len - 1];
int tail_y = s->y[s->len - 1];
for (i = s->len - 1; i > 0; i--) {
s->x[i] = s->x[i - 1];
s->y[i] = s->y[i - 1];
}
switch (s->dir) {
case UP:
s->y[0]--;
break;
case RIGHT:
s->x[0]++;
break;
case DOWN:
s->y[0]++;
break;
case LEFT:
s->x[0]--;
break;
}
if (game[s->y[0]][s->x[0]] == '@') {
s->len++;
generate_food(food);
(*score)++;
} else if (game[s->y[0]][s->x[0]] == 'O' || game[s->y[0]][s->x[0]] == '#') {
printf("Game over!\n");
exit(0);
}
game[tail_y][tail_x] = ' ';
game[s->y[0]][s->x[0]] = 'O';
}
// 检测是否按下方向键并改变蛇的方向
void check_direction(struct Snake *s) {
if (_kbhit()) {
switch (_getch()) {
case 'w':
case 'W':
case 72:
if (s->dir != DOWN) {
s->dir = UP;
}
break;
case 'd':
case 'D':
case 77:
if (s->dir != LEFT) {
s->dir = RIGHT;
}
break;
case 's':
case 'S':
case 80:
if (s->dir != UP) {
s->dir = DOWN;
}
break;
case 'a':
case 'A':
case 75:
if (s->dir != RIGHT) {
s->dir = LEFT;
}
break;
}
}
}
int main() {
int score = 0;
struct Snake snake;
struct Food food;
init_game();
init_snake(&snake);
generate_food(&food);
while (1) {
system("cls");
draw_game();
printf("Score: %d\n", score);
move_snake(&snake, &food, &score);
check_direction(&snake);
Sleep(100);
}
return 0;
}
```
这个游戏是在 Windows 系统下使用 Visual Studio 进行开发的,如果要在其他平台上运行,可能需要做一些适当的修改。
相关推荐
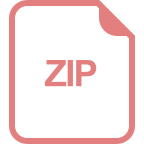
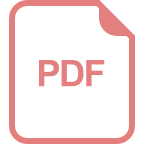
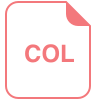
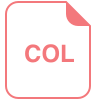
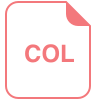
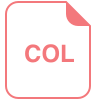
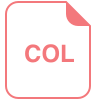


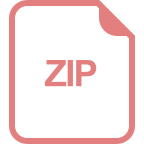
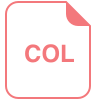
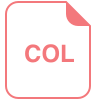
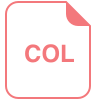
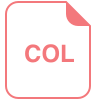
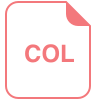