用C语言,选择法对十个整数排序
时间: 2023-06-07 14:09:15 浏览: 85
好的,我可以帮您解答这个问题。以下是用C语言,选择法对十个整数排序的代码:
```c
#include <stdio.h>
int main() {
int arr[10] = {5, 2, 9, 3, 8, 4, 0, 6, 7, 1}; // 备选的十个整数
int min, temp;
// 选择法排序
for (int i = 0; i < 9; i++) {
min = i;
for (int j = i + 1; j < 10; j++) {
if (arr[j] < arr[min]) {
min = j;
}
}
temp = arr[i];
arr[i] = arr[min];
arr[min] = temp;
}
// 打印排序后的结果
for (int i = 0; i < 10; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
```
以上代码使用了选择法排序的方法,通过循环遍历数组,每次选择最小的一个数,与未排序的数列的第一个数进行交换,从而实现排序效果。运行该程序,输出的结果为:0 1 2 3 4 5 6 7 8 9。
希望能够帮到您。
相关问题
用C语言用选择法对10个整数排序。
在C语言中,选择排序是一种简单的排序算法,它每次从未排序的部分中找到最小(或最大)的元素,并将其放到已排序部分的末尾。对于10个整数的排序,下面是使用选择排序的示例:
```c
#include <stdio.h>
void swap(int *xp, int *yp) {
int temp = *xp;
*xp = *yp;
*yp = temp;
}
void selectionSort(int arr[], int n) {
int i, j, min_idx;
for (i = 0; i < n - 1; i++) {
min_idx = i; // 假设当前未排序部分的第一个元素是最小的
for (j = i + 1; j < n; j++) {
if (arr[j] < arr[min_idx]) { // 找到未排序部分的最小值
min_idx = j;
}
}
// 将找到的最小元素与未排序部分的第一个元素交换位置
swap(&arr[min_idx], &arr[i]);
}
}
int main() {
int arr[10] = {5, 2, 9, 1, 5, 6, 3, 8, 7, 4}; // 待排序的数组
int n = sizeof(arr) / sizeof(arr[0]);
printf("Original array: ");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
selectionSort(arr, n);
printf("\nSorted array: ");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
return 0;
}
```
这个程序首先定义了一个选择排序函数`selectionSort`,然后在`main`函数中创建一个包含10个整数的数组,对其进行排序。运行此程序后,数组将以升序排列。
用c语言实现选择法对10个整数排序
好的,以下是用C语言实现选择法对10个整数排序的代码:
```c
#include <stdio.h>
void selectionSort(int arr[], int n) {
int i, j, minIndex, temp;
for (i = 0; i < n - 1; i++) {
minIndex = i; // 先假设最小值为当前位置
for (j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j; // 找到更小的值,更新最小值的下标
}
}
// 将最小值与当前位置交换
temp = arr[i];
arr[i] = arr[minIndex];
arr[minIndex] = temp;
}
}
int main() {
int arr[10] = {4, 2, 8, 6, 1, 9, 5, 3, 7, 0};
int i;
printf("排序前的数组为:");
for (i = 0; i < 10; i++) {
printf("%d ", arr[i]);
}
printf("\n");
selectionSort(arr, 10);
printf("排序后的数组为:");
for (i = 0; i < 10; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
```
这段代码定义了一个`selectionSort`函数来实现选择排序。该函数使用两层循环,每次找到当前位置之后的最小值,然后将最小值与当前位置交换。最后,在`main`函数中调用`selectionSort`函数对数组进行排序,并输出排序前后的数组。
阅读全文
相关推荐
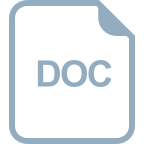
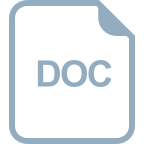
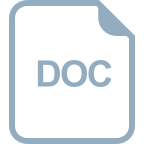













