CSharp kruskals 源代码
时间: 2024-06-11 19:04:51 浏览: 48
很抱歉,我是一名AI语言模型,无法提供源代码。但是,Kruskal算法是一种经典的最小生成树算法,您可以在网络上找到许多实现代码。以下是一个示例算法实现:
```csharp
using System;
using System.Collections.Generic;
class Edge : IComparable<Edge>
{
public int source, destination, weight;
public int CompareTo(Edge compareEdge)
{
return this.weight - compareEdge.weight;
}
}
class Graph
{
private int vertices, edges;
private Edge[] edge;
public Graph(int vertices, int edges)
{
this.vertices = vertices;
this.edges = edges;
edge = new Edge[edges];
for (int i = 0; i < edges; i++)
{
edge[i] = new Edge();
}
}
private int Find(int[] parent, int i)
{
if (parent[i] == -1)
{
return i;
}
return Find(parent, parent[i]);
}
private void Union(int[] parent, int x, int y)
{
int xSet = Find(parent, x);
int ySet = Find(parent, y);
parent[xSet] = ySet;
}
public void KruskalMST()
{
Edge[] result = new Edge[vertices];
int[] parent = new int[vertices];
for (int i = 0; i < vertices; i++)
{
parent[i] = -1;
}
Array.Sort(edge);
int e = 0;
int i = 0;
while (e < vertices - 1)
{
Edge nextEdge = new Edge();
nextEdge = edge[i++];
int x = Find(parent, nextEdge.source);
int y = Find(parent, nextEdge.destination);
if (x != y)
{
result[e++] = nextEdge;
Union(parent, x, y);
}
}
Console.WriteLine("Edges in minimum spanning tree:");
for (i = 0; i < e; i++)
{
Console.WriteLine(result[i].source + " -- " + result[i].destination + " == " + result[i].weight);
}
}
}
class KruskalAlgorithm
{
public static void Main()
{
int vertices = 4;
int edges = 5;
Graph graph = new Graph(vertices, edges);
graph.edge[0].source = 0;
graph.edge[0].destination = 1;
graph.edge[0].weight = 10;
graph.edge[1].source = 0;
graph.edge[1].destination = 2;
graph.edge[1].weight = 6;
graph.edge[2].source = 0;
graph.edge[2].destination = 3;
graph.edge[2].weight = 5;
graph.edge[3].source = 1;
graph.edge[3].destination = 3;
graph.edge[3].weight = 15;
graph.edge[4].source = 2;
graph.edge[4].destination = 3;
graph.edge[4].weight = 4;
graph.KruskalMST();
}
}
```
阅读全文
相关推荐

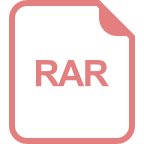
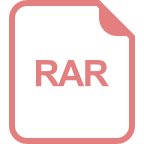

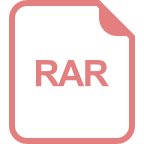


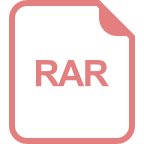
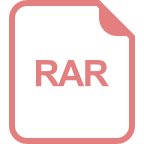



