双向RRT※算法MATLAB
时间: 2023-08-02 20:08:34 浏览: 297
您好!双向RRT(Rapidly-exploring Random Tree)算法是一种用于路径规划的算法,它通过两个随机生成的树(一棵从起点开始,另一棵从终点开始)来搜索可行路径。下面是一个使用MATLAB实现双向RRT算法的示例代码:
```matlab
function [path, tree] = bidirectionalRRT(start, goal, obstacles, mapSize, maxIter, stepSize)
% 初始化树的节点
tree.start.pos = start;
tree.start.parent = 0;
tree.goal.pos = goal;
tree.goal.parent = 0;
% 迭代搜索
for i = 1:maxIter
% 在起点树上随机采样一个点
if rand < 0.5
randPos = rand(1, 2) .* mapSize;
[nearestNode, ~] = nearestNeighbor(tree.start, randPos);
newNode.pos = steer(nearestNode.pos, randPos, stepSize);
newNode.parent = nearestNode;
% 检查新节点是否与障碍物相交
if ~collisionCheck(newNode.pos, nearestNode.pos, obstacles)
tree.start = addNode(tree.start, newNode);
tree.start = rewire(tree.start, newNode, stepSize);
% 检查新节点是否与终点树的节点相交
[goalNode, dist] = nearestNeighbor(tree.goal, newNode.pos);
if ~collisionCheck(newNode.pos, goalNode.pos, obstacles)
path = getPath(tree.start, newNode);
path = [path; flip(getPath(tree.goal, goalNode))];
return;
end
end
else
% 在终点树上随机采样一个点,过程与起点树相似
randPos = rand(1, 2) .* mapSize;
[nearestNode, ~] = nearestNeighbor(tree.goal, randPos);
newNode.pos = steer(nearestNode.pos, randPos, stepSize);
newNode.parent = nearestNode;
if ~collisionCheck(newNode.pos, nearestNode.pos, obstacles)
tree.goal = addNode(tree.goal, newNode);
tree.goal = rewire(tree.goal, newNode, stepSize);
[startNode, dist] = nearestNeighbor(tree.start, newNode.pos);
if ~collisionCheck(newNode.pos, startNode.pos, obstacles)
path = getPath(tree.start, startNode);
path = [path; flip(getPath(tree.goal, newNode))];
return;
end
end
end
end
path = [];
tree = [];
end
function [nearestNode, minDist] = nearestNeighbor(tree, pos)
nearestNode = tree;
minDist = norm(pos - tree.pos);
% 寻找最近的节点
queue = tree;
while ~isempty(queue)
node = queue(1);
queue(1) = [];
dist = norm(pos - node.pos);
if dist < minDist
minDist = dist;
nearestNode = node;
end
queue = [queue node.children];
end
end
function newPos = steer(fromPos, toPos, stepSize)
direction = toPos - fromPos;
distance = norm(direction);
if distance <= stepSize
newPos = toPos;
else
newPos = fromPos + direction / distance * stepSize;
end
end
function tree = addNode(tree, newNode)
newNode.children = [];
tree.children = [tree.children newNode];
end
function tree = rewire(tree, newNode, maxDist)
queue = newNode.children;
while ~isempty(queue)
node = queue(1);
queue(1) = [];
dist = norm(newNode.pos - node.pos);
if dist <= maxDist
node.parent = newNode;
queue = [queue node.children];
end
end
end
function collides = collisionCheck(pos1, pos2, obstacles)
collides = false;
for i = 1:size(obstacles, 1)
if lineSegmentIntersectsPolygon(pos1, pos2, obstacles{i})
collides = true;
return;
end
end
end
function intersects = lineSegmentIntersectsPolygon(pos1, pos2, polygon)
intersects = false;
for i = 1:size(polygon, 1)
pos3 = polygon(i, :);
pos4 = polygon(mod(i, size(polygon, 1)) + 1, :);
if lineSegmentsIntersect(pos1, pos2, pos3, pos4)
intersects = true;
return;
end
end
end
function intersects = lineSegmentsIntersect(pos1, pos2, pos3, pos4)
intersects = false;
p1 = pos1';
p2 = pos2';
q1 = pos3';
q2 = pos4';
r = p2 - p1;
s = q2 - q1;
uNumerator = cross(q1 - p1, r);
denominator = cross(r, s);
if denominator == 0
if uNumerator == 0
intersects = true;
end
return;
end
u = uNumerator / denominator;
t = cross(q1 - p1, s) / denominator;
if t >= 0 && t <= 1 && u >= 0 && u <= 1
intersects = true;
end
end
function path = getPath(tree, node)
path = [];
while node.parent ~= 0
path = [path; node.pos];
node = node.parent;
end
path = [path; tree.start.pos];
end
```
这段代码实现了双向RRT算法,其中`start`和`goal`表示起点和终点的坐标,`obstacles`表示障碍物的坐标,`mapSize`表示地图的尺寸,`maxIter`表示最大迭代次数,`stepSize`表示每次扩展的步长。函数返回的`path`是搜索到的路径,`tree`是搜索得到的树。
请注意,这只是一个简单的实现示例,您可能需要根据自己的具体需求进行适当的修改和优化。希望对您有帮助!
阅读全文
相关推荐
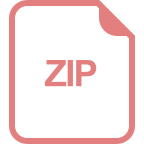
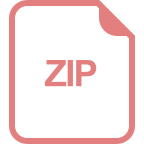








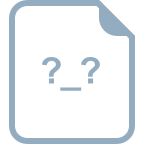
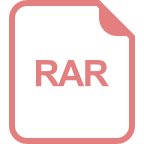
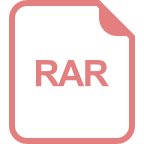
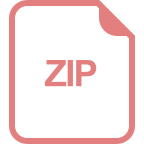