怎么将相机拍出的照片传送到编辑页面,编辑页面照片和文本框,点击save按钮后封装成note保存到Room数据库,点击cancel按钮,返回相机拍照界面,并且删除该照片,完整代码
时间: 2023-10-03 13:02:18 浏览: 66
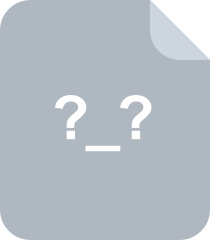
拍照上传到数据库
以下是一个实现该功能的完整代码:
首先,在布局文件中添加一个相机预览和一个保存/取消按钮:
```xml
<RelativeLayout
android:id="@+id/camera_preview"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextureView
android:id="@+id/texture_view"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
<Button
android:id="@+id/btn_save"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Save"
android:layout_alignParentBottom="true"
android:layout_alignParentStart="true"/>
<Button
android:id="@+id/btn_cancel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Cancel"
android:layout_alignParentBottom="true"
android:layout_alignParentEnd="true"/>
</RelativeLayout>
```
然后,在相机预览页面中,设置 TextureView 来预览相机拍摄的画面,并且获取相机权限:
```java
public class CameraPreviewActivity extends AppCompatActivity implements TextureView.SurfaceTextureListener {
private Camera mCamera;
private TextureView mTextureView;
private Button mBtnSave;
private Button mBtnCancel;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_camera_preview);
mTextureView = findViewById(R.id.texture_view);
mTextureView.setSurfaceTextureListener(this);
mBtnSave = findViewById(R.id.btn_save);
mBtnSave.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
saveNote();
}
});
mBtnCancel = findViewById(R.id.btn_cancel);
mBtnCancel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
cancelNote();
}
});
}
@Override
public void onSurfaceTextureAvailable(SurfaceTexture surface, int width, int height) {
mCamera = Camera.open();
try {
mCamera.setPreviewTexture(surface);
mCamera.startPreview();
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void onSurfaceTextureSizeChanged(SurfaceTexture surface, int width, int height) {
// Ignored, Camera does all the work for us
}
@Override
public boolean onSurfaceTextureDestroyed(SurfaceTexture surface) {
mCamera.stopPreview();
mCamera.release();
return true;
}
@Override
public void onSurfaceTextureUpdated(SurfaceTexture surface) {
// Invoked every time there's a new Camera preview frame
}
private void saveNote() {
// 将照片和文本框封装成 Note 对象,并保存到 Room 数据库中
}
private void cancelNote() {
// 删除照片,并返回相机拍照界面
File photoFile = new File(getExternalFilesDir(null), "photo.jpg");
if (photoFile.exists()) {
photoFile.delete();
}
Intent intent = new Intent(this, MainActivity.class);
startActivity(intent);
finish();
}
}
```
在 saveNote 方法中,将照片和文本框封装成 Note 对象,并保存到 Room 数据库中:
```java
private void saveNote() {
EditText editText = findViewById(R.id.edit_text);
String text = editText.getText().toString();
File photoFile = new File(getExternalFilesDir(null), "photo.jpg");
Note note = new Note();
note.setText(text);
note.setPhotoPath(photoFile.getAbsolutePath());
AppDatabase.getDatabase(this).noteDao().insert(note);
Intent intent = new Intent(this, MainActivity.class);
startActivity(intent);
finish();
}
```
在 cancelNote 方法中,删除照片,并返回相机拍照界面:
```java
private void cancelNote() {
File photoFile = new File(getExternalFilesDir(null), "photo.jpg");
if (photoFile.exists()) {
photoFile.delete();
}
Intent intent = new Intent(this, MainActivity.class);
startActivity(intent);
finish();
}
```
最后,在相机拍照界面中,启动相机预览页面:
```java
public class MainActivity extends AppCompatActivity {
private static final int REQUEST_IMAGE_CAPTURE = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btnTakePhoto = findViewById(R.id.btn_take_photo);
btnTakePhoto.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
dispatchTakePictureIntent();
}
});
}
private void dispatchTakePictureIntent() {
Intent takePictureIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
if (takePictureIntent.resolveActivity(getPackageManager()) != null) {
File photoFile = null;
try {
photoFile = createImageFile();
} catch (IOException ex) {
ex.printStackTrace();
}
if (photoFile != null) {
Uri photoURI = FileProvider.getUriForFile(this, "com.example.android.fileprovider", photoFile);
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
startActivityForResult(takePictureIntent, REQUEST_IMAGE_CAPTURE);
}
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_IMAGE_CAPTURE && resultCode == RESULT_OK) {
Intent intent = new Intent(this, CameraPreviewActivity.class);
startActivity(intent);
finish();
}
}
private File createImageFile() throws IOException {
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
String imageFileName = "JPEG_" + timeStamp + "_";
File storageDir = getExternalFilesDir(null);
File image = File.createTempFile(
imageFileName, /* prefix */
".jpg", /* suffix */
storageDir /* directory */
);
return image;
}
}
```
在 onActivityResult 方法中,启动相机预览页面,如果拍摄成功,则会返回 RESULT_OK,否则不做任何处理。
createImageFile 方法用于创建一个临时文件来存储拍摄的照片。
注意,在 AndroidManifest.xml 文件中需要添加以下权限和 provider:
```xml
<uses-permission android:name="android.permission.CAMERA"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<provider
android:name="androidx.core.content.FileProvider"
android:authorities="com.example.android.fileprovider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths"/>
</provider>
```
其中,file_paths.xml 文件中定义了 FileProvider 的路径:
```xml
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-files-path name="my_images" path="Pictures" />
</paths>
```
阅读全文
相关推荐
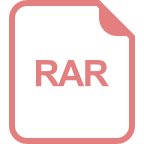
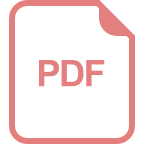


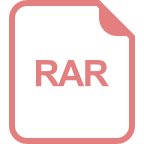
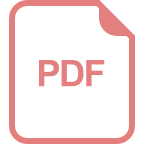
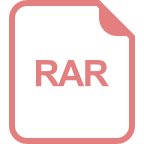
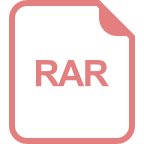
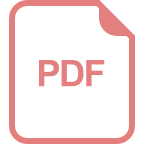
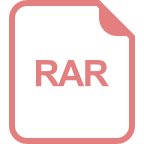
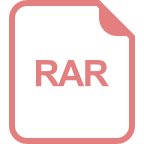
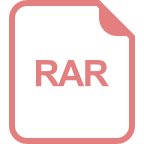
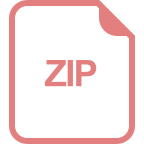
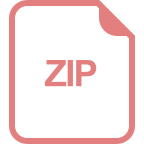
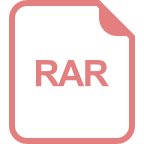
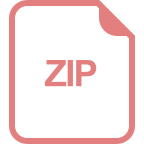