用Python写出读取testData目录中存储的数字符号数据文件(每个数字有1个存储文件,命名规则与训练数据相同),通过模型对符号进行分类预测并分别输出分类正确和错误的数字。提示:如果模型预测结果与文件名中第一部分存储的数字相同代表预测正确;汉明距离计算可用第三方Levenshtein 模块,from Levenshtein import hamming,hamming('0010', '1111')结果为3。
时间: 2024-02-28 14:53:24 浏览: 44
好的,可以通过以下步骤来实现:
1. 首先需要加载模型,可以使用以下代码:
```python
from transformers import AutoTokenizer, AutoModelForSequenceClassification, pipeline
# 加载 tokenizer
tokenizer = AutoTokenizer.from_pretrained("ckpt/tokenizer")
# 加载模型
model = AutoModelForSequenceClassification.from_pretrained("ckpt/model")
classifier = pipeline('text-classification', model=model, tokenizer=tokenizer)
```
2. 然后需要读取测试数据目录中的文件,并对每个文件进行分类预测,可以使用以下代码:
```python
import os
from Levenshtein import hamming
test_data_dir = 'testData'
correct_count = 0
wrong_count = 0
for filename in os.listdir(test_data_dir):
# 读取文件内容
filepath = os.path.join(test_data_dir, filename)
with open(filepath, 'r') as f:
text = f.read().strip()
# 预测分类
result = classifier(text)[0]
predicted_label = result['label']
true_label = filename.split('_')[0]
# 判断预测结果是否正确
if predicted_label == true_label:
correct_count += 1
else:
wrong_count += 1
# 计算汉明距离
distance = hamming(predicted_label, true_label)
print(f'预测错误:文件名={filename},预测结果={predicted_label},真实标签={true_label},汉明距离={distance}')
print(f'分类正确的数量:{correct_count}')
print(f'分类错误的数量:{wrong_count}')
```
这段代码会遍历测试数据目录中的所有文件,读取文件内容,然后使用模型进行分类预测。如果预测结果与文件名中的数字相同,则认为预测结果是正确的;否则认为预测结果是错误的,并计算预测结果与真实标签之间的汉明距离。最后输出分类正确和错误的数字的数量。
阅读全文
相关推荐
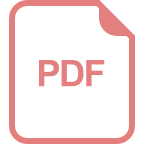
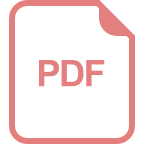
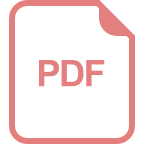















