C# 客户端有上传下载按钮,点击上传,选择文件,然后文件存储到服务器端的mysql数据库中,点击下载,选择MySQL数据库中的文件,下载到本地,可以选择本地路径
时间: 2024-02-27 09:56:18 浏览: 15
好的,你的问题是如何用 C# 实现客户端上传和下载文件到 MySQL 数据库中?
首先,你需要连接到 MySQL 数据库。你可以使用 MySQL Connector/NET,它是一个用 C# 编写的 MySQL 数据库连接库。
然后,你可以使用以下代码来上传文件到 MySQL 数据库中:
```csharp
using MySql.Data.MySqlClient;
using System.IO;
// 连接到 MySQL 数据库
string connectionString = "server=localhost;user=root;database=mydb;password=mypassword;";
using (MySqlConnection connection = new MySqlConnection(connectionString))
{
connection.Open();
// 选择要上传的文件
string filePath = "C:\\path\\to\\file.txt";
string fileName = Path.GetFileName(filePath);
// 读取文件内容
byte[] fileContent = File.ReadAllBytes(filePath);
// 将文件内容插入到 MySQL 数据库中
string sql = "INSERT INTO files (name, content) VALUES (@name, @content)";
using (MySqlCommand command = new MySqlCommand(sql, connection))
{
command.Parameters.AddWithValue("@name", fileName);
command.Parameters.AddWithValue("@content", fileContent);
command.ExecuteNonQuery();
}
}
```
其中,`files` 是 MySQL 数据库中的表名,`name` 和 `content` 分别是文件名和文件内容的列名。
接下来,你可以使用以下代码来从 MySQL 数据库中下载文件:
```csharp
using MySql.Data.MySqlClient;
using System.IO;
// 连接到 MySQL 数据库
string connectionString = "server=localhost;user=root;database=mydb;password=mypassword;";
using (MySqlConnection connection = new MySqlConnection(connectionString))
{
connection.Open();
// 选择要下载的文件
string fileName = "file.txt";
// 从 MySQL 数据库中读取文件内容
string sql = "SELECT content FROM files WHERE name = @name";
using (MySqlCommand command = new MySqlCommand(sql, connection))
{
command.Parameters.AddWithValue("@name", fileName);
using (MySqlDataReader reader = command.ExecuteReader())
{
if (reader.Read())
{
byte[] fileContent = (byte[])reader["content"];
// 保存文件到本地
string savePath = "C:\\path\\to\\save\\file.txt";
File.WriteAllBytes(savePath, fileContent);
}
}
}
}
```
其中,`file.txt` 是要下载的文件名,`savePath` 是要保存到本地的文件路径。
希望这些代码可以帮助你实现客户端上传和下载文件到 MySQL 数据库中。
相关推荐
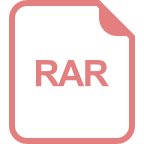
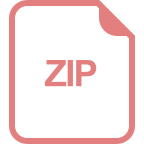
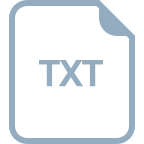














