前端vue大文件切片上传 代码
时间: 2023-08-07 16:08:24 浏览: 106
以下是一个基于Vue.js的大文件切片上传的示例代码:
```
<template>
<div>
<input type="file" ref="fileInput" @change="onFileChange">
<button @click="upload">上传</button>
</div>
</template>
<script>
export default {
data() {
return {
file: null,
chunkSize: 1024 * 1024, // 切片大小,这里设置为1M
chunks: [], // 切片数组
uploadedChunks: [] // 已上传的切片数组
}
},
methods: {
onFileChange(event) {
this.file = event.target.files[0]
},
async upload() {
// 分割文件为切片
await this.splitFile()
// 上传所有切片
await this.uploadChunks()
// 合并所有切片
await this.mergeChunks()
},
async splitFile() {
const fileSize = this.file.size
let offset = 0
while (offset < fileSize) {
const chunk = await this.readChunk(offset, this.chunkSize)
this.chunks.push(chunk)
offset += this.chunkSize
}
},
async readChunk(offset, length) {
return new Promise((resolve, reject) => {
const reader = new FileReader()
reader.onload = event => {
resolve(event.target.result)
}
reader.onerror = error => {
reject(error)
}
const blob = this.file.slice(offset, offset + length)
reader.readAsArrayBuffer(blob)
})
},
async uploadChunks() {
for (let i = 0; i < this.chunks.length; i++) {
const chunk = this.chunks[i]
if (this.uploadedChunks.includes(i)) {
// 如果该切片已上传,则跳过
continue
}
await this.uploadChunk(chunk, i)
}
},
async uploadChunk(chunk, index) {
// 使用axios或其他http库上传切片
// 每次上传切片时,需要将该切片的索引和总切片数一并上传
// 服务器需要记录已上传的切片索引,以便后续合并文件
},
async mergeChunks() {
// 合并所有切片,生成最终的文件
// 合并方式可以是使用Blob对象的arrayBuffer()和new Blob()方法
// 也可以是在服务器端合并
// 合并后的文件可以直接下载或上传到云存储
}
}
}
</script>
```
以上代码仅为示例,具体的切片上传实现方式需要根据具体场景进行调整。同时,上传切片和合并文件的具体实现需要与后端服务器进行配合。
阅读全文
相关推荐
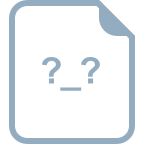
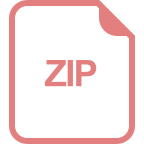
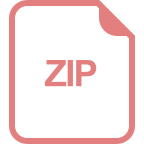





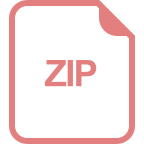

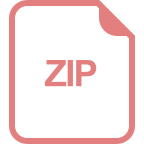
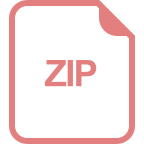
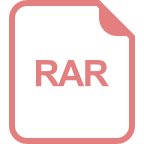
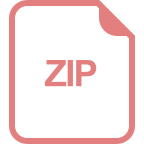
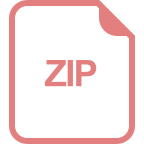
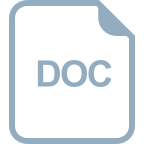
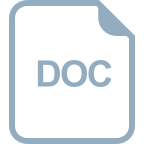
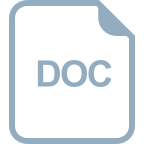