c语言编程使用消息队列从父进程发送10个整数到子进程,子进程收下来后计算总和,后用同一个消息队列把总和发回给父进程显示用循环逐个元素发送
时间: 2023-05-29 16:04:56 浏览: 92
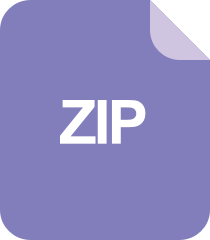
C实现的一个简单的循环消息队列
以下是一个简单的实现:
父进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/msg.h>
#include <sys/ipc.h>
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
int mtext[10];
};
int main() {
int msgid;
key_t key = 1234;
struct msgbuf message;
int sum = 0;
// 创建消息队列
msgid = msgget(key, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 发送消息
for (int i = 0; i < 10; i++) {
message.mtype = 1;
message.mtext[i] = i + 1;
if (msgsnd(msgid, &message, sizeof(message.mtext), 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
}
// 接收消息
if (msgrcv(msgid, &message, sizeof(message.mtext), 2, 0) == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
for (int i = 0; i < 10; i++) {
sum += message.mtext[i];
}
printf("Sum: %d\n", sum);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
```
子进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/msg.h>
#include <sys/ipc.h>
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
int mtext[10];
};
int main() {
int msgid;
key_t key = 1234;
struct msgbuf message;
int sum = 0;
// 获取消息队列
msgid = msgget(key, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 接收消息
if (msgrcv(msgid, &message, sizeof(message.mtext), 1, 0) == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
for (int i = 0; i < 10; i++) {
sum += message.mtext[i];
}
// 发送消息
message.mtype = 2;
for (int i = 0; i < 10; i++) {
message.mtext[i] = 0;
}
message.mtext[0] = sum;
if (msgsnd(msgid, &message, sizeof(message.mtext), 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
```
阅读全文
相关推荐
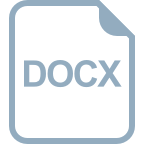


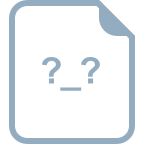
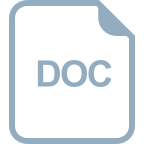
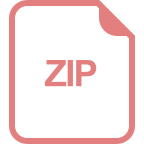
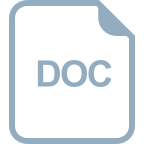
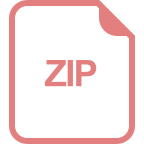
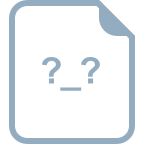
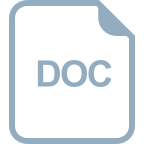
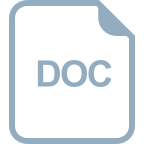
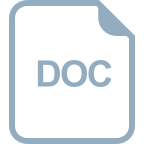
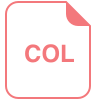