spring boot 定时任务设置结束时间
时间: 2023-08-16 20:05:23 浏览: 128
在 Spring Boot 中,可以使用 @Scheduled 注解来实现定时任务。如果需要设置结束时间,可以使用 ScheduledFuture 类型的返回值来控制任务的执行和取消。
具体实现步骤如下:
1. 在定时任务方法上添加 @Scheduled 注解,并设置 cron 表达式或 fixedRate、fixedDelay 等属性。
2. 在定时任务方法内部,使用 ScheduledExecutorService 的 schedule 方法来执行任务,并将返回值赋值给 ScheduledFuture 类型的变量。
3. 在需要结束任务的时候,调用 ScheduledFuture 的 cancel 方法,将任务取消。
以下是一个示例代码:
```java
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.ScheduledThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
@Component
public class MyTask {
private ScheduledFuture<?> future;
@Scheduled(cron = "0/10 * * * * ?") // 每隔 10 秒执行一次
public void run() {
if (future == null) {
ScheduledExecutorService executorService = new ScheduledThreadPoolExecutor(1);
future = executorService.schedule(() -> {
// 任务逻辑
}, 30, TimeUnit.SECONDS); // 任务执行时间为 30 秒
}
}
public void stop() {
if (future != null) {
future.cancel(true);
future = null;
}
}
}
```
在上面的代码中,定时任务方法 run 中使用了 ScheduledExecutorService 的 schedule 方法来执行任务,并将返回值赋值给 ScheduledFuture 类型的变量 future。在 stop 方法中,调用 future 的 cancel 方法来取消任务。
阅读全文
相关推荐
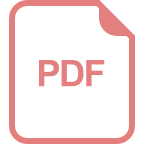
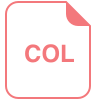
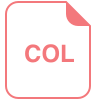
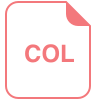
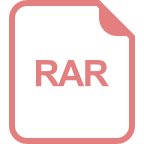
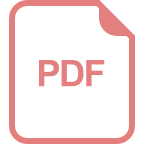
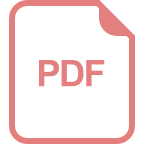
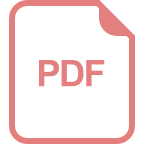
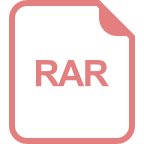
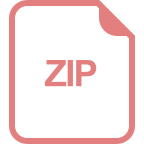
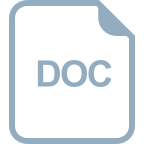
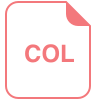
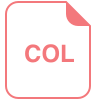
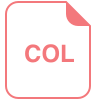
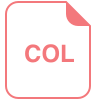
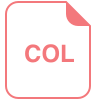