可以举个例子吗? 增加资源开销的例子
时间: 2024-03-27 07:36:50 浏览: 54
下面是一个增加资源开销的例子,使用 move 语义的开销比使用拷贝构造函数的开销更小:
```cpp
#include <iostream>
#include <vector>
#include <chrono>
using namespace std;
using namespace std::chrono;
struct MyStruct {
int id;
double score;
vector<int>* data;
MyStruct() : id(0), score(0.0), data(nullptr) {}
~MyStruct() { delete data; }
MyStruct(const MyStruct& other) {
id = other.id;
score = other.score;
data = new vector<int>(*other.data);
}
MyStruct& operator=(const MyStruct& other) {
if (this != &other) {
id = other.id;
score = other.score;
delete data;
data = new vector<int>(*other.data);
}
return *this;
}
MyStruct(MyStruct&& other) {
id = other.id;
score = other.score;
data = other.data;
other.data = nullptr;
}
MyStruct& operator=(MyStruct&& other) {
if (this != &other) {
id = other.id;
score = other.score;
delete data;
data = other.data;
other.data = nullptr;
}
return *this;
}
};
int main() {
MyStruct a{1, 90.5, new vector<int>{1,2,3,4,5}};
auto start1 = high_resolution_clock::now();
MyStruct b = move(a); // 使用移动构造函数
auto end1 = high_resolution_clock::now();
auto duration1 = duration_cast<microseconds>(end1 - start1);
cout << "使用移动构造函数的时间:" << duration1.count() << " 微秒" << endl;
MyStruct c{2, 85.5, new vector<int>{6,7,8,9,10}};
auto start2 = high_resolution_clock::now();
MyStruct d = c; // 使用拷贝构造函数
auto end2 = high_resolution_clock::now();
auto duration2 = duration_cast<microseconds>(end2 - start2);
cout << "使用拷贝构造函数的时间:" << duration2.count() << " 微秒" << endl;
return 0;
}
```
在上面的例子中,我们重新定义了包含一个指向 vector 类型对象的指针成员变量的 struct 类型 `MyStruct`。我们重载了拷贝构造函数和赋值运算符函数,以实现对指针成员变量的深拷贝操作。
在进行移动操作时,我们只需要将原对象中的指针成员变量赋值给新对象,而不需要重新分配和复制指针指向的内存。因此,使用移动构造函数的开销要比使用拷贝构造函数的开销更小。
需要注意的是,在使用移动语义时,原对象中的指针成员变量会被移动到新对象中,因此在移动操作后,原对象中的指针成员变量会变为 nullptr,不能再被使用。
阅读全文
相关推荐
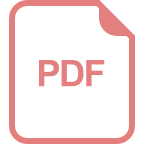
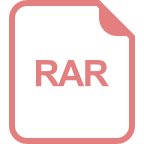
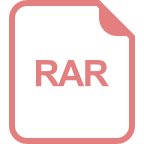
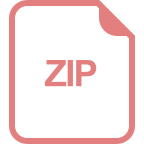
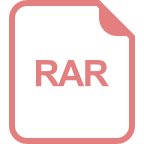
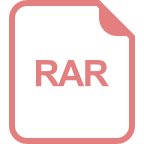
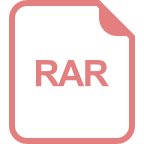
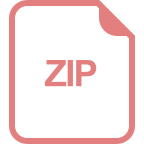
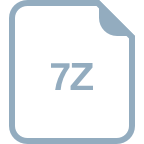
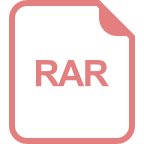
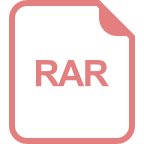
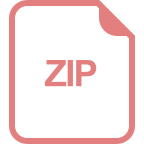
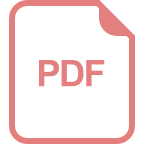
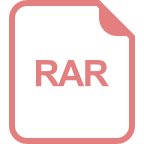
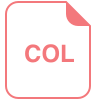


