easyexcel 导出合并行和列的表头的excel
时间: 2023-10-25 10:00:08 浏览: 168
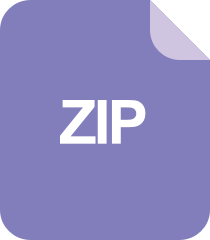
Java导出excel工具详细介绍(POI 和 EasyExcel),各种复杂需求情况的导出(包括动态设置合并单元格等)

EasyExcel 支持导出合并行和列的表头的 Excel,可以通过设置 `@HeadRowHeight`、`@HeadColumnWidth`、`@ContentRowHeight` 和 `@ContentColumnWidth` 注解来控制表头和内容的行高和列宽。同时,可以通过设置 `@ExcelProperty` 注解的 `colspan` 和 `rowspan` 属性来合并行和列。
以下是一个例子,导出一个合并了表头的 Excel:
```java
@ExcelIgnoreUnannotated
public class ExportData {
@ExcelProperty(index = 0, value = "姓名", rowspan = 2, colspan = 2)
@HeadRowHeight(30)
@HeadColumnWidth(15)
private String name;
@ExcelProperty(index = 2, value = "性别", rowspan = 2)
@HeadRowHeight(30)
@HeadColumnWidth(15)
private String gender;
@ExcelProperty(index = 3, value = "联系方式", colspan = 2)
@HeadRowHeight(30)
@HeadColumnWidth(20)
private String contact;
@ExcelProperty(index = 4, value = "电话")
@HeadRowHeight(30)
@HeadColumnWidth(15)
private String phone;
@ExcelProperty(index = 5, value = "邮箱")
@HeadRowHeight(30)
@HeadColumnWidth(20)
private String email;
// 其他属性...
// getter/setter 方法...
}
```
在这个例子中,我们使用 `@HeadRowHeight` 和 `@HeadColumnWidth` 注解设置表头的行高和列宽,使用 `@ContentRowHeight` 和 `@ContentColumnWidth` 注解设置内容的行高和列宽。同时,我们使用 `@ExcelProperty` 注解的 `colspan` 和 `rowspan` 属性来合并行和列。注意,需要将 `@ExcelIgnoreUnannotated` 注解添加到类上,以忽略未注解的属性。
然后,我们可以使用 EasyExcel 的 `ExcelWriter` 和 `Sheet` 类来写入数据。以下是一个示例代码:
```java
public class ExcelExportUtil {
public static void exportExcel(List<ExportData> dataList, String fileName, HttpServletResponse response) throws IOException {
// 设置响应头
response.setContentType("application/vnd.ms-excel");
response.setCharacterEncoding("utf-8");
response.setHeader("Content-disposition", "attachment;filename=" + fileName + ".xlsx");
// 创建 ExcelWriter
ServletOutputStream outputStream = response.getOutputStream();
ExcelWriter excelWriter = new ExcelWriter(outputStream, ExcelTypeEnum.XLSX);
// 创建 Sheet
Sheet sheet = new Sheet(1, 0, ExportData.class);
sheet.setTableStyle(createTableStyle());
// 写入数据
excelWriter.write(dataList, sheet);
// 关闭 ExcelWriter
excelWriter.finish();
}
private static TableStyle createTableStyle() {
TableStyle tableStyle = new TableStyle();
tableStyle.setTableContentBackGroundColor(IndexedColors.WHITE);
tableStyle.setTableContentFontName("宋体");
tableStyle.setTableContentFontSize(12);
tableStyle.setTableHeadBackGroundColor(IndexedColors.GREY_25_PERCENT);
tableStyle.setTableHeadFontName("宋体");
tableStyle.setTableHeadFontSize(14);
tableStyle.setTableHeadFontBold(true);
tableStyle.setTableHeadFontColor(IndexedColors.WHITE);
return tableStyle;
}
}
```
在这个例子中,我们使用 `ExcelWriter` 和 `Sheet` 类来写入数据,使用 `createTableStyle()` 方法创建表格样式。注意,需要设置表格样式,否则导出的 Excel 可能会出现样式问题。
最后,在需要导出 Excel 的方法中调用 `ExcelExportUtil.exportExcel()` 方法即可。例如:
```java
public class ExportController {
@GetMapping("/export")
public void export(HttpServletResponse response) throws IOException {
List<ExportData> dataList = // 查询数据...
ExcelExportUtil.exportExcel(dataList, "export", response);
}
}
```
阅读全文
相关推荐
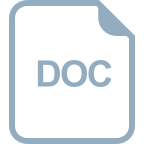
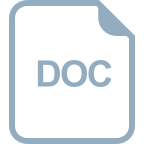
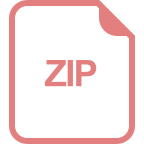












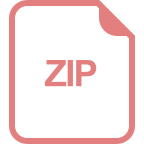

