Given two strings, s1 and s2, write a program to return a new string made of s1 and s2’s first, middle, and last characters.
时间: 2024-10-11 09:16:38 浏览: 22
为了编写一个程序,将两个字符串`s1`和`s2`的第一个、中间和最后一个字符组合成一个新的字符串,你可以按照以下步骤操作。这里我会提供一个Python示例:
```python
def combine_strings(s1, s2):
# 检查字符串长度,避免在计算中间字符时出错
if len(s1) == 0 or len(s2) == 0:
return s1[0] + s2[0]
# 计算中间字符的位置
mid_index = (len(s1) - 1) // 2
# 如果s1比s2长,取s2的全部字符作为新的字符串
if len(s1) > len(s2):
result = s1[0] + s2 + s1[mid_index]
else:
# 否则,如果s2比s1长,取s1的全部字符并在最后加上s2的中间字符
result = s1 + s2[:mid_index + 1]
# 添加结束字符
result += s1[-1] if len(s1) > len(s2) else s2[-1]
return result
# 测试函数
s1 = "abcdef"
s2 = "ghijkl"
new_string = combine_strings(s1, s2)
print(new_string)
```
这个函数首先检查两个字符串的长度,然后确定需要取哪个字符串的中间字符。之后,它将三个部分拼接起来形成新的字符串,并在末尾添加各自最末尾的一个字符。
阅读全文
相关推荐
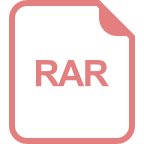
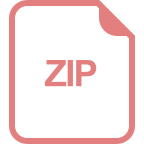
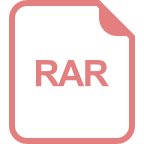

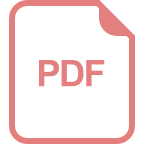
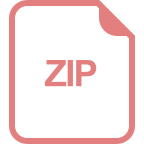
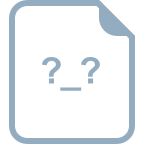
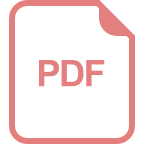
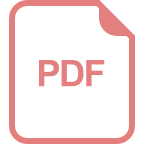
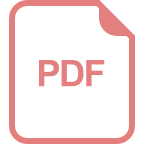
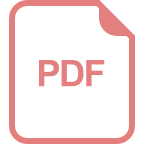
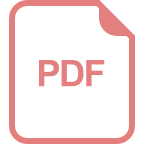
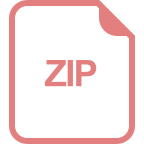
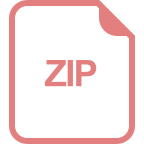
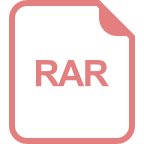
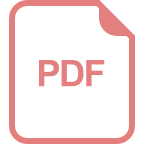
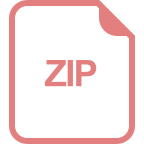