超市商品管理系统c++
时间: 2023-06-29 08:10:47 浏览: 88
超市商品管理系统可以分为以下模块:
1. 商品信息管理模块:包括商品的分类、名称、价格、库存等信息的录入、修改、删除、查询等功能。
2. 销售管理模块:包括销售单的生成、修改、删除等功能,以及销售统计分析功能。
3. 进货管理模块:包括进货单的生成、修改、删除等功能,以及进货统计分析功能。
4. 库存管理模块:包括商品库存的增加、减少、盘点等功能。
下面是一个简单的超市商品管理系统的C++代码示例:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 商品类
class Goods {
public:
string name; // 商品名称
string category; // 商品分类
double price; // 商品价格
int stock; // 商品库存
Goods(string name = "", string category = "", double price = 0.0, int stock = 0) {
this->name = name;
this->category = category;
this->price = price;
this->stock = stock;
}
void display() { // 显示商品信息
cout << "商品名称:" << name << endl;
cout << "商品分类:" << category << endl;
cout << "商品价格:" << price << endl;
cout << "商品库存:" << stock << endl;
}
};
// 商品管理类
class GoodsManager {
private:
vector<Goods> goodsList; // 商品列表
public:
// 添加商品
void addGoods(Goods goods) {
goodsList.push_back(goods);
cout << "添加商品成功!" << endl;
}
// 删除商品
void deleteGoods(string name) {
for (int i = 0; i < goodsList.size(); i++) {
if (goodsList[i].name == name) {
goodsList.erase(goodsList.begin() + i);
cout << "删除商品成功!" << endl;
return;
}
}
cout << "未找到该商品!" << endl;
}
// 修改商品信息
void modifyGoods(string name) {
for (int i = 0; i < goodsList.size(); i++) {
if (goodsList[i].name == name) {
cout << "请输入新的商品名称:";
string newName;
cin >> newName;
cout << "请输入新的商品分类:";
string newCategory;
cin >> newCategory;
cout << "请输入新的商品价格:";
double newPrice;
cin >> newPrice;
cout << "请输入新的商品库存:";
int newStock;
cin >> newStock;
goodsList[i] = Goods(newName, newCategory, newPrice, newStock);
cout << "修改商品成功!" << endl;
return;
}
}
cout << "未找到该商品!" << endl;
}
// 查询商品信息
void queryGoods(string name) {
for (int i = 0; i < goodsList.size(); i++) {
if (goodsList[i].name == name) {
goodsList[i].display();
return;
}
}
cout << "未找到该商品!" << endl;
}
// 显示所有商品信息
void displayAll() {
for (int i = 0; i < goodsList.size(); i++) {
goodsList[i].display();
cout << endl;
}
}
};
int main() {
GoodsManager goodsManager;
int choice;
while (true) {
cout << "1. 添加商品" << endl;
cout << "2. 删除商品" << endl;
cout << "3. 修改商品信息" << endl;
cout << "4. 查询商品信息" << endl;
cout << "5. 显示所有商品信息" << endl;
cout << "6. 退出" << endl;
cout << "请输入你的选择:";
cin >> choice;
switch (choice) {
case 1: {
cout << "请输入商品名称:";
string name;
cin >> name;
cout << "请输入商品分类:";
string category;
cin >> category;
cout << "请输入商品价格:";
double price;
cin >> price;
cout << "请输入商品库存:";
int stock;
cin >> stock;
Goods goods(name, category, price, stock);
goodsManager.addGoods(goods);
break;
}
case 2: {
cout << "请输入商品名称:";
string name;
cin >> name;
goodsManager.deleteGoods(name);
break;
}
case 3: {
cout << "请输入要修改的商品名称:";
string name;
cin >> name;
goodsManager.modifyGoods(name);
break;
}
case 4: {
cout << "请输入要查询的商品名称:";
string name;
cin >> name;
goodsManager.queryGoods(name);
break;
}
case 5: {
goodsManager.displayAll();
break;
}
case 6: {
return 0;
}
default: {
cout << "输入有误,请重新输入!" << endl;
break;
}
}
}
return 0;
}
```
这是一个简单的示例,实际的超市商品管理系统还需要更多功能和细节的处理。
阅读全文
相关推荐

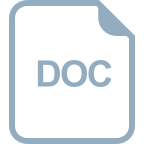
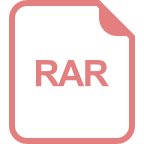
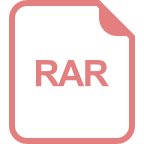

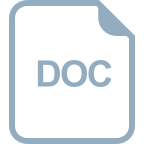
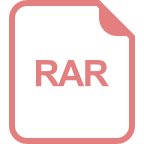
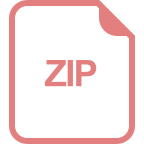


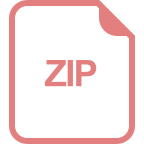
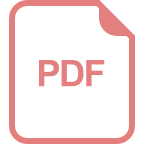