写一个linux c语言线程池小程序并详细解释该程序
时间: 2023-04-07 18:04:58 浏览: 126
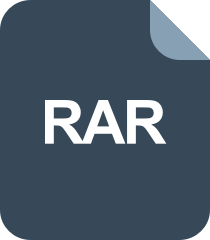
用c写的一个关于线程池的代码

好的,我可以回答这个问题。线程池是一种常用的并发编程技术,它可以提高程序的性能和可维护性。下面是一个简单的 Linux C 语言线程池小程序的实现:
首先,我们需要定义一个任务结构体,用于存储任务的信息:
```
typedef struct {
void (*function)(void *); // 任务函数指针
void *argument; // 任务参数
} task_t;
```
然后,我们需要定义一个线程池结构体,用于存储线程池的信息:
```
typedef struct {
pthread_mutex_t lock; // 互斥锁
pthread_cond_t notify; // 条件变量
pthread_t *threads; // 线程数组
task_t *queue; // 任务队列
int thread_count; // 线程数量
int queue_size; // 任务队列大小
int head; // 队头
int tail; // 队尾
int count; // 任务数量
int shutdown; // 关闭标志
} threadpool_t;
```
接下来,我们需要实现线程池的初始化函数:
```
int threadpool_init(threadpool_t *pool, int thread_count, int queue_size) {
// 初始化互斥锁和条件变量
pthread_mutex_init(&pool->lock, NULL);
pthread_cond_init(&pool->notify, NULL);
// 初始化线程池信息
pool->threads = (pthread_t *)malloc(thread_count * sizeof(pthread_t));
pool->queue = (task_t *)malloc(queue_size * sizeof(task_t));
pool->thread_count = thread_count;
pool->queue_size = queue_size;
pool->head = pool->tail = pool->count = 0;
pool->shutdown = 0;
// 创建线程
for (int i = 0; i < thread_count; i++) {
pthread_create(&pool->threads[i], NULL, threadpool_worker, (void *)pool);
}
return 0;
}
```
然后,我们需要实现线程池的销毁函数:
```
int threadpool_destroy(threadpool_t *pool) {
// 设置关闭标志
pool->shutdown = 1;
// 唤醒所有线程
pthread_cond_broadcast(&pool->notify);
// 等待所有线程退出
for (int i = 0; i < pool->thread_count; i++) {
pthread_join(pool->threads[i], NULL);
}
// 释放线程池信息
free(pool->threads);
free(pool->queue);
// 销毁互斥锁和条件变量
pthread_mutex_destroy(&pool->lock);
pthread_cond_destroy(&pool->notify);
return 0;
}
```
接下来,我们需要实现线程池的任务添加函数:
```
int threadpool_add_task(threadpool_t *pool, void (*function)(void *), void *argument) {
// 获取互斥锁
pthread_mutex_lock(&pool->lock);
// 判断任务队列是否已满
if (pool->count == pool->queue_size) {
pthread_mutex_unlock(&pool->lock);
return -1;
}
// 添加任务到队尾
pool->queue[pool->tail].function = function;
pool->queue[pool->tail].argument = argument;
pool->tail = (pool->tail + 1) % pool->queue_size;
pool->count++;
// 唤醒一个线程
pthread_cond_signal(&pool->notify);
// 释放互斥锁
pthread_mutex_unlock(&pool->lock);
return 0;
}
```
最后,我们需要实现线程池的工作线程函数:
```
void *threadpool_worker(void *arg) {
threadpool_t *pool = (threadpool_t *)arg;
while (1) {
// 获取互斥锁
pthread_mutex_lock(&pool->lock);
// 等待任务或关闭标志
while (pool->count == 0 && !pool->shutdown) {
pthread_cond_wait(&pool->notify, &pool->lock);
}
// 判断是否需要退出
if (pool->shutdown) {
pthread_mutex_unlock(&pool->lock);
pthread_exit(NULL);
}
// 取出任务
void (*function)(void *) = pool->queue[pool->head].function;
void *argument = pool->queue[pool->head].argument;
pool->head = (pool->head + 1) % pool->queue_size;
pool->count--;
// 释放互斥锁
pthread_mutex_unlock(&pool->lock);
// 执行任务
function(argument);
}
return NULL;
}
```
以上就是一个简单的 Linux C 语言线程池小程序的实现。线程池的主要思想是将任务添加到队列中,然后由工作线程从队列中取出任务并执行。线程池可以提高程序的性能和可维护性,特别是在处理大量并发任务时。
阅读全文
相关推荐
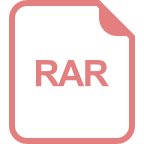
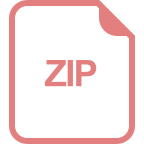
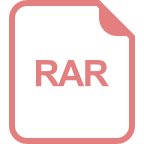
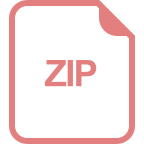
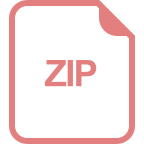
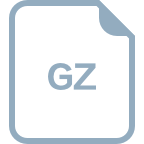
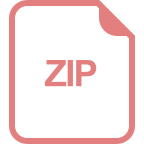
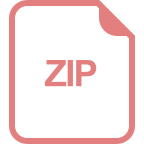
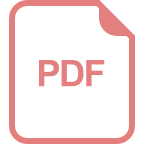
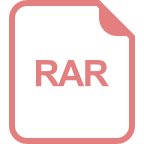
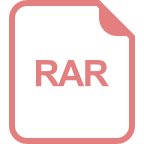
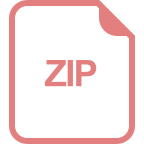
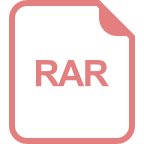
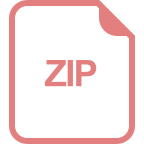
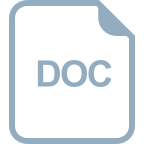
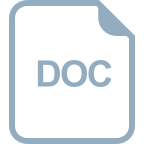
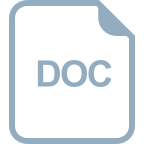