打开摄像头,实时识别二维码(或打开本地视频文件,识别二维码) 要求:在视频中容许一定的二维码几何畸变而且二维码几何矫正 用Python编程
时间: 2024-05-08 07:18:27 浏览: 13
好的,关于打开摄像头实时识别二维码的问题,你可以使用Python中的OpenCV库和zbar库实现。
以下是实现步骤:
1. 安装OpenCV和zbar库,你可以使用pip工具进行安装。
2. 导入相关库:
```python
import cv2
from pyzbar import pyzbar
```
3. 打开摄像头:
```python
cam = cv2.VideoCapture(0)
```
下面介绍如何对视频文件进行处理。首先需要导入视频文件:
```python
cam = cv2.VideoCapture("videoFile.mp4")
```
4. 读取视频帧:
```python
_, frame = cam.read()
```
5. 将图像转换为灰度图像:
```python
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
```
6. 使用zbar库对二维码进行解码:
```python
barcodes = pyzbar.decode(gray)
```
7. 显示图像和解码结果:
```python
for barcode in barcodes:
(x, y, w, h) = barcode.rect
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 0, 255), 2)
barcodeData = barcode.data.decode("utf-8")
barcodeType = barcode.type
cv2.putText(frame, barcodeData, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 2)
print("Barcode Type: {}, Barcode Data: {}".format(barcodeType, barcodeData))
cv2.imshow("Barcode Scanner", frame)
```
完整代码如下所示:
```python
import cv2
from pyzbar import pyzbar
cam = cv2.VideoCapture(0)
while True:
_, frame = cam.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
barcodes = pyzbar.decode(gray)
for barcode in barcodes:
(x, y, w, h) = barcode.rect
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 0, 255), 2)
barcodeData = barcode.data.decode("utf-8")
barcodeType = barcode.type
cv2.putText(frame, barcodeData, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 2)
print("Barcode Type: {}, Barcode Data: {}".format(barcodeType, barcodeData))
cv2.imshow("Barcode Scanner", frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cam.release()
cv2.destroyAllWindows()
```
这样,你就可以通过打开摄像头或者打开视频文件实时识别二维码并做一定几何矫正了。
相关推荐
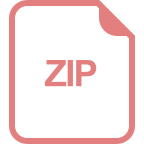














