springmvc和elementui
时间: 2023-08-26 15:06:25 浏览: 99
Spring MVC 是一个 Java web 框架,用于构建基于 MVC(模型-视图-控制器)架构的 Web 应用程序。它提供了一个丰富的功能集,包括路由、控制器、视图解析器、数据绑定、表单验证等等。
Element UI 是一个基于 Vue.js 的 UI 组件库,提供了一系列常用的 UI 组件,如按钮、表格、日期选择器等。它具有美观易用的特点,同时也支持自定义主题。
这两个技术栈在开发 Web 应用时可以相互配合使用。Spring MVC 可以作为后端框架提供数据接口,而 Element UI 可以作为前端 UI 组件库进行页面开发。通过 Ajax 请求,前端与后端可以进行数据交互,从而实现一体化的 Web 应用。
相关问题
写一下springmvc + vue的大文件分片上传代码。要求:1.前端加入MD5;2.用elementui
好的,以下是一个示例代码,使用SpringMVC和Vue实现大文件分片上传,同时前端加入MD5校验,使用ElementUI实现进度条显示。
前端代码:
```vue
<template>
<div>
<el-upload
class="upload-demo"
action="/upload"
:before-upload="beforeUpload"
:on-progress="onProgress"
:on-success="onSuccess"
:file-list="fileList">
<el-button type="primary">点击上传</el-button>
<div slot="tip" class="el-upload__tip">只能上传不超过 200MB 的文件</div>
</el-upload>
<el-progress :percentage="percentage" :stroke-width="30" :text-inside="true"></el-progress>
</div>
</template>
<script>
import SparkMD5 from 'spark-md5'
export default {
data() {
return {
fileList: [],
percentage: 0,
}
},
methods: {
beforeUpload(file) {
return new Promise((resolve, reject) => {
const reader = new FileReader()
const chunkSize = 2 * 1024 * 1024 // 分片大小为2MB
const chunks = Math.ceil(file.size / chunkSize)
const spark = new SparkMD5.ArrayBuffer()
let currentChunk = 0
reader.onload = e => {
spark.append(e.target.result)
currentChunk++
if (currentChunk < chunks) {
loadNext()
} else {
file.md5 = spark.end()
resolve(file)
}
}
reader.onerror = () => {
reject('读取文件出错')
}
function loadNext() {
const start = currentChunk * chunkSize
const end = Math.min(start + chunkSize, file.size)
reader.readAsArrayBuffer(file.slice(start, end))
}
loadNext()
})
},
onProgress(e) {
this.percentage = e.percent
},
onSuccess(response, file) {
this.percentage = 0
this.fileList = []
this.$message.success('上传成功')
},
},
}
</script>
```
在这个示例代码中,我们使用了SparkMD5库来计算文件的MD5,同时在`beforeUpload`方法中,将文件分成多个2MB的分片进行上传,以实现大文件分片上传和MD5校验。在上传过程中,我们使用了ElementUI的进度条组件来显示上传进度。
后端代码:
```java
@Controller
public class UploadController {
private static final String UPLOAD_DIR = "/tmp/uploads/";
private static final int CHUNK_SIZE = 2 * 1024 * 1024;
@RequestMapping(value = "/upload", method = RequestMethod.POST)
@ResponseBody
public ResponseEntity<?> upload(@RequestParam("file") MultipartFile file, @RequestParam("md5") String md5,
@RequestParam(value = "chunk", required = false, defaultValue = "0") int chunk,
@RequestParam(value = "chunks", required = false, defaultValue = "1") int chunks) throws IOException {
String fileName = file.getOriginalFilename();
String filePath = UPLOAD_DIR + fileName;
if (chunk == 0) {
// 如果是第一个分片,检查文件是否存在,如果存在则直接返回
File f = new File(filePath);
if (f.exists()) {
return ResponseEntity.status(HttpStatus.OK).build();
}
} else {
// 如果不是第一个分片,检查文件是否存在,如果不存在则返回错误
File f = new File(filePath);
if (!f.exists()) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("文件不存在");
}
}
if (chunk == 0) {
// 如果是第一个分片,创建文件
File f = new File(filePath);
if (!f.getParentFile().exists()) {
f.getParentFile().mkdirs();
}
f.createNewFile();
}
// 写入分片数据
RandomAccessFile raf = new RandomAccessFile(filePath, "rw");
raf.seek(chunk * CHUNK_SIZE);
raf.write(file.getBytes());
raf.close();
if (chunk == chunks - 1) {
// 如果是最后一个分片,检查文件MD5值是否正确,如果正确则合并文件
String fileMd5 = getFileMd5(filePath);
if (!fileMd5.equals(md5)) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("文件MD5值不正确");
}
mergeFileChunks(filePath, chunks);
}
return ResponseEntity.status(HttpStatus.OK).build();
}
private String getFileMd5(String filePath) throws IOException {
InputStream fis = new FileInputStream(filePath);
byte[] buffer = new byte[1024];
int numRead;
MessageDigest md5;
try {
md5 = MessageDigest.getInstance("MD5");
} catch (NoSuchAlgorithmException e) {
return null;
}
do {
numRead = fis.read(buffer);
if (numRead > 0) {
md5.update(buffer, 0, numRead);
}
} while (numRead != -1);
fis.close();
byte[] md5Bytes = md5.digest();
StringBuilder sb = new StringBuilder();
for (byte b : md5Bytes) {
sb.append(Integer.toString((b & 0xff) + 0x100, 16).substring(1));
}
return sb.toString();
}
private void mergeFileChunks(String filePath, int chunks) throws IOException {
File f = new File(filePath);
FileOutputStream fos = new FileOutputStream(f, true);
byte[] buffer = new byte[1024];
for (int i = 0; i < chunks; i++) {
String chunkFilePath = filePath + "." + i;
File chunk = new File(chunkFilePath);
FileInputStream fis = new FileInputStream(chunk);
int numRead;
while ((numRead = fis.read(buffer)) > 0) {
fos.write(buffer, 0, numRead);
}
fis.close();
chunk.delete();
}
fos.close();
}
}
```
在后端代码中,我们使用了`RandomAccessFile`来实现文件的分片写入和合并,同时在文件合并前使用了`getFileMd5`方法来检查文件的MD5值是否正确。
最后,我们还需要在SpringMVC的配置文件中添加以下配置,以支持大文件上传:
```xml
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="maxUploadSize" value="209715200" />
<property name="maxInMemorySize" value="4096" />
</bean>
```
阅读全文
相关推荐
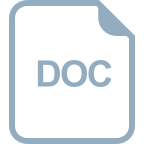
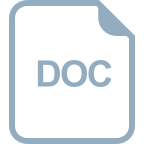
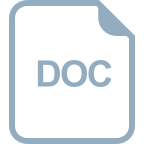
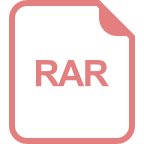
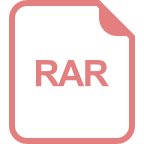
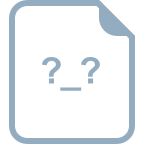
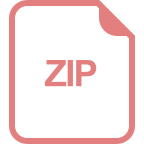
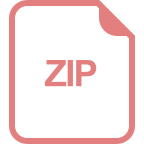
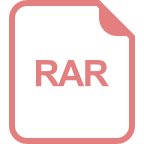
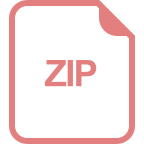
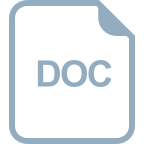
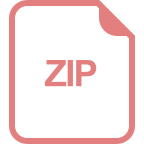
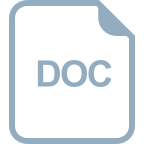
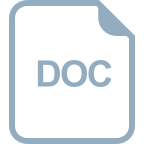
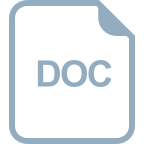
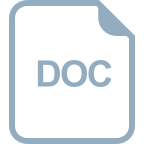
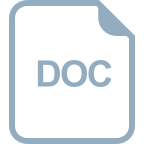
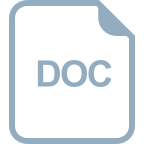