matlab 异或置乱代码
时间: 2024-09-17 11:08:10 浏览: 78
MATLAB中异或置乱(XOR scrambling)是一种数据加密技术,用于保护数据安全。它通过将原始数据与一个随机产生的二进制序列(通常是一个预先设定的密钥)进行按位异或操作,使得数据变得难以识别。以下是简单的异或置乱代码示例:
```matlab
% 假设原始数据(需要加密)
data = [1 2 3 4 5];
% 随机生成的密钥(假设长度与数据相等)
key = randi([0 1], length(data), 1); % 生成0或1的随机数
% 异或置乱过程
scrambled_data = data xor key; % 按位异或操作
% 显示原数据和混淆后的数据
disp("Original Data:");
disp(data);
disp("Scrambled Data (XOR scrambled):");
disp(scrambled_data);
% 如果需要还原数据,只需同样进行一次异或操作,因为异或是可逆的
decrypted_data = scrambled_data xor key;
disp("Decrypted Data:");
disp(decrypted_data);
```
注意,这只是一个基本示例,实际应用中可能还需要考虑密钥的安全管理和存储。此外,MATLAB本身并不直接支持高级加密算法,上述代码仅适用于简单演示。
相关问题
matlab实现图像先置乱再加密的代码
以下是用Matlab实现图像先置乱再加密的示例代码:
```matlab
% 图像先置乱再加密
% 读取图像
image = imread('input_image.jpg');
% 图像置乱(使用随机置换)
[row, col, ~] = size(image);
shuffled_image = zeros(row, col, 3);
permuted_indices = randperm(row * col);
for i = 1 : 3
channel = image(:, :, i);
shuffled_channel = channel(permuted_indices);
shuffled_image(:, :, i) = reshape(shuffled_channel, row, col);
end
% 加密(使用简单异或操作)
key = randi([0, 255], row, col, 3, 'uint8');
encrypted_image = bitxor(shuffled_image, key);
% 显示原始图像、置乱后的图像和加密后的图像
figure;
subplot(1, 3, 1);
imshow(image);
title('原始图像');
subplot(1, 3, 2);
imshow(shuffled_image);
title('置乱后的图像');
subplot(1, 3, 3);
imshow(encrypted_image);
title('加密后的图像');
% 保存加密后的图像
imwrite(encrypted_image, 'encrypted_image.jpg');
```
请注意,这只是一个简单的示例代码,用于说明图像先置乱再加密的过程。实际应用中,可能需要更复杂的算法和更强的加密方法来确保安全性。
基于混沌的图像置乱加密算法及matlab的实现,(完整版)基于MATLAB的混沌序列图像加密程序...
混沌序列在图像加密中广泛应用,因为它们能够生成高度随机的序列,这些序列可以用作密钥或加密过程中的伪随机数。
以下是一个基于MATLAB的混沌序列图像加密程序的简单实现:
1. 生成混沌序列
混沌序列是通过迭代某些非线性方程得到的。在这个例子中,我们将使用 Logistic 映射方程:
$x_{n+1} = r x_n (1 - x_n)$
其中 $x_n$ 是当前状态,$x_{n+1}$ 是下一个状态,$r$ 是一个控制参数。我们选择一个适当的初始值 $x_0$,并迭代 $N$ 次以生成一个长度为 $N$ 的混沌序列。
以下是 MATLAB 代码:
```matlab
function y = logisticmap(x0, r, N)
% Generates a chaotic sequence using the logistic map equation
% x_n+1 = r * x_n * (1 - x_n)
% x0: initial value
% r: control parameter
% N: number of iterations
x = zeros(N, 1);
x(1) = x0;
for i = 2:N
x(i) = r * x(i-1) * (1 - x(i-1));
end
y = x;
end
```
2. 图像置乱
我们可以使用混沌序列来置乱图像像素的顺序。具体来说,我们将像素按照混沌序列的顺序重新排列。
以下是 MATLAB 代码:
```matlab
function y = shuffleimage(x, seq)
% Shuffles the pixels of an image using a chaotic sequence
% x: input image
% seq: chaotic sequence
[M, N] = size(x);
y = zeros(M, N);
% Reshape the image into a vector
xvec = reshape(x, [], 1);
% Shuffle the vector using the chaotic sequence
xvec = xvec(seq);
% Reshape the shuffled vector into an image
y(:) = xvec;
end
```
3. 图像加密
使用混沌序列对图像进行加密需要两个步骤:图像置乱和像素混淆。我们已经介绍了图像置乱,现在我们需要实现像素混淆。
对于像素混淆,我们将使用一个简单的异或操作。具体来说,我们将混沌序列中的每个元素与像素值进行异或运算。这种方法可以提供一定的保护,但不足以防止高级攻击。
以下是 MATLAB 代码:
```matlab
function y = encryptimage(x, r, x0)
% Encrypts an image using a chaotic sequence
% x: input image
% r: control parameter of the logistic map
% x0: initial value of the logistic map
seq = logisticmap(x0, r, numel(x));
shuffled = shuffleimage(x, seq);
% XOR the shuffled image with the chaotic sequence
y = bitxor(shuffled, uint8(seq));
end
```
现在我们可以将这些函数组合起来,生成一个完整的图像加密程序:
```matlab
x = imread('lena.png');
r = 4.0;
x0 = 0.1;
y = encryptimage(x, r, x0);
imshow(y);
```
这个程序将读取名为 “lena.png” 的图像,使用 $r=4.0$ 和 $x_0=0.1$ 作为参数进行加密,并显示加密后的图像。注意,我们使用了 MATLAB 内置的 `imread` 和 `imshow` 函数来读取和显示图像。
这只是一个简单的例子,实际的图像加密需要更复杂的算法和更高的安全性。
阅读全文
相关推荐
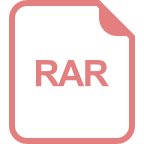
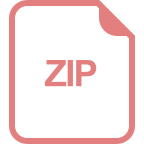
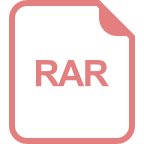
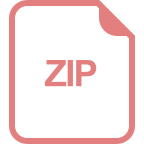
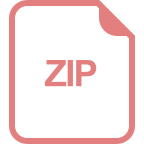
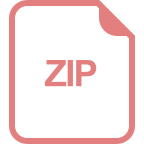
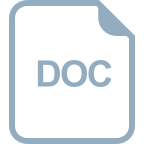
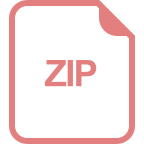
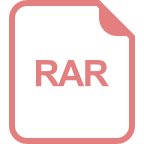
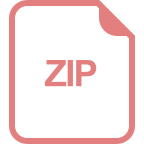
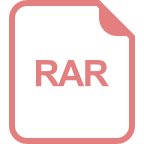
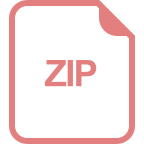
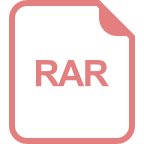
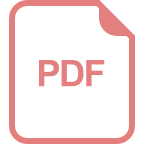
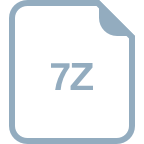
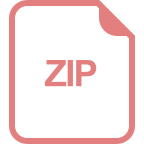