java中如何使用steam流更改指定项目的值
时间: 2024-12-11 22:45:20 浏览: 11
在Java中,Stream API提供了一种强大的方式来处理集合数据,但它本身并不直接支持修改集合元素的操作。如果你想通过Stream对集合中的项目(例如Map、List或其他支持修改的集合)进行值的更新,你需要结合其他操作。
对于Map,你可以使用`map()`函数创建一个新的Map,然后用`putAll()`方法合并到原Map中:
```java
Map<String, Integer> originalMap = ...; // 原始地图
int newValue = ...; // 新的值
// 使用Stream更改值
Map<String, Integer> updatedMap = originalMap.entrySet()
.stream() // 转换成流
.collect(Collectors.toMap(
Map.Entry::getKey,
entry -> (entry.getValue() == oldValue) ? newValue : entry.getValue(), // 更新规则
(oldValue, newValue) -> oldValue)); // 合并策略,这里默认保留旧值
updatedMap.putAll(originalMap); // 将更改后的流合回原始Map
```
对于List,可以先筛选出需要改变的元素,然后使用`forEach`遍历并更新它们:
```java
List<Integer> originalList = ...; // 原始列表
int newValue = ...; // 新的值
int oldValue = ...;
originalList.stream()
.filter(item -> item == oldValue) // 筛选出需要替换的元素
.forEach(item -> item = newValue); // 更新元素值
// 或者创建新的List,如果不需要保留原始顺序
List<Integer> updatedList = originalList.stream()
.distinct() // 如果需要去重
.replaceAllMatch(oldValue, newValue)
.collect(Collectors.toList());
```
请注意,上述代码示例假设`oldValue`在原始集合中存在,如果不是这样,你可能需要添加错误处理。另外,`replaceAllMatch`是Java 9及更高版本引入的API。
阅读全文
相关推荐
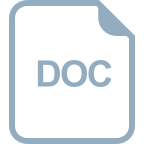
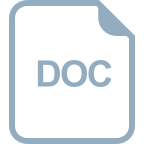
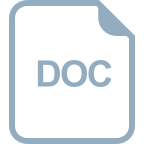


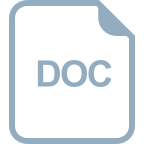
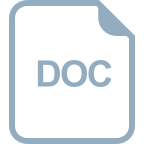
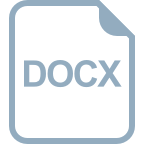
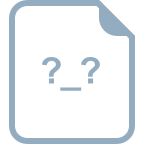
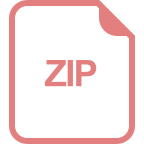
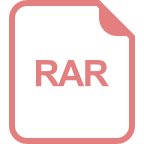
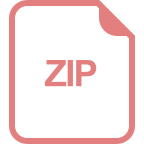