python 维吉尼亚密码的代码(不需要规定为大写字母)
时间: 2024-09-13 12:14:39 浏览: 22
维吉尼亚密码(Vigenère cipher)是一种使用简单多表代换的加密方法,它利用一个关键字对明文进行加密。每个字母都根据关键字中对应位置的字母来进行移位,关键字会重复使用,直到覆盖整个明文。这里提供一个简单的Python实现示例:
```python
def vigenere_encrypt(plaintext, keyword):
alphabet = 'abcdefghijklmnopqrstuvwxyz'
key_length = len(keyword)
plaintext = plaintext.lower()
keyword = keyword.lower()
ciphertext = ''
for i in range(len(plaintext)):
if plaintext[i].isalpha():
p_index = alphabet.index(plaintext[i])
k_index = alphabet.index(keyword[i % key_length])
c_index = (p_index + k_index) % 26
ciphertext += alphabet[c_index]
else:
ciphertext += plaintext[i]
return ciphertext
def vigenere_decrypt(ciphertext, keyword):
alphabet = 'abcdefghijklmnopqrstuvwxyz'
key_length = len(keyword)
ciphertext = ciphertext.lower()
keyword = keyword.lower()
plaintext = ''
for i in range(len(ciphertext)):
if ciphertext[i].isalpha():
c_index = alphabet.index(ciphertext[i])
k_index = alphabet.index(keyword[i % key_length])
p_index = (c_index - k_index) % 26
plaintext += alphabet[p_index]
else:
plaintext += ciphertext[i]
return plaintext
# 示例使用
plaintext = "hello world"
keyword = "python"
encrypted = vigenere_encrypt(plaintext, keyword)
print("Encrypted text:", encrypted)
decrypted = vigenere_decrypt(encrypted, keyword)
print("Decrypted text:", decrypted)
```
这段代码包含了两个函数,`vigenere_encrypt` 用于加密,`vigenere_decrypt` 用于解密。它们都接受明文和关键字作为参数,并返回处理后的密文或解密后的明文。
请注意,这个实现只考虑了小写字母,并且没有过滤掉非字母字符。如果需要处理大写字母,可以适当调整`alphabet`字符串以包含大写字母,并在处理时转换大小写。
相关推荐
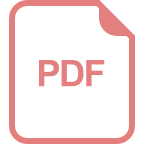
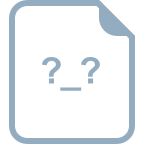
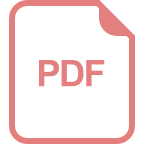














