知道一个月有多少天,知道第一天是星期几,利用c语言写一段代码,打印出当月日历表
时间: 2024-11-09 09:29:32 浏览: 27
在C语言中,我们可以使用标准库中的`<ctime>`和`<stdio.h>`来实现这个功能。以下是一个基础的例子,它会打印出指定月份的日历,并包含星期几和月份的名称。请注意,这个例子假设用户输入的是有效年份和月份。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
// 定义一个结构体存储日期信息
struct Date {
int day;
char weekday[10];
};
// 函数用于获取给定日期的星期几
void get_weekday(struct Date *date) {
time_t now = time(0);
tm *ltm = localtime(&now);
ltm->tm_mday = date->day;
// 格式化得到星期几
strftime(date->weekday, sizeof(date->weekday), "%A", ltm);
}
// 主函数
int main() {
int year, month;
printf("Enter the year and month (format: YYYY MM): ");
scanf("%d %d", &year, &month);
// 检查月份是否合法
if(month < 1 || month > 12) {
printf("Invalid month.\n");
return 1;
}
// 初始化月份的日历
struct Date calendar[32]; // 一般月份最多有31天,闰年2月最多29天
calendar[0].day = 1;
calendar[0].weekday[0] = '\0'; // 初始化为空,稍后填充
// 计算星期几并填充日历
for (int i = 1; i <= 31; i++) {
calendar[i].day = i;
get_weekday(&calendar[i]);
}
// 打印日历
printf("%s %d, %d\n", calendar[0].weekday, calendar[0].day, year);
printf("----------------------------------------\n");
for (int i = 0; i < 7; i++) {
for (int j = 0; j <= 31 && j >= i; j++) {
printf("%2d ", calendar[j].day);
}
printf("\n");
}
printf("----------------------------------------\n");
return 0;
}
```
阅读全文
相关推荐
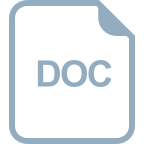
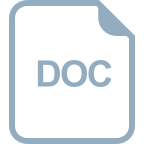
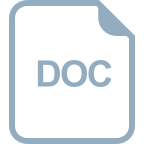
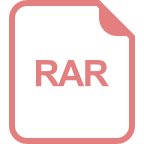
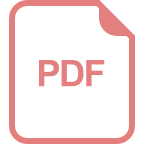
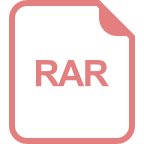
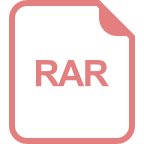
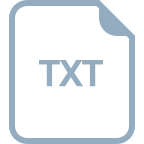
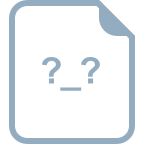
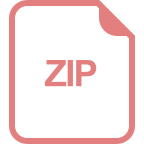
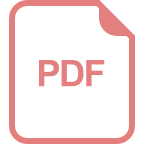