C# try catch MESSAHE BOX SHOW
时间: 2024-08-07 17:00:56 浏览: 62
在C#中,`try-catch`块用于处理程序执行过程中的异常情况,保证程序不会因为错误就终止。当一段代码可能会抛出异常(例如除数为零、文件读取失败等),你可以将其放在`try`部分。如果这个代码块内部发生了预期之外的异常,C#会立即跳转到相应的`catch`块中执行。
`MessageBox.Show`是一个Windows Forms中的API函数,它会在控制台上显示一个消息框,向用户报告错误信息。在`catch`块中,通常你会创建一个`MessageBox`实例,并传递错误消息给它,比如这样:
```csharp
try
{
// 可能引发异常的代码
}
catch (Exception ex)
{
MessageBox.Show("发生错误:" + ex.Message, "错误", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
```
这里,`ex.Message`获取的是异常的具体描述信息,然后显示在一个包含"错误"标题的消息框中,用户可以看到并采取相应措施。` MessageBoxButtons.OK`表示单击按钮关闭对话框,`MessageBoxIcon.Error`设置图标为警告样式。
相关问题
string json = File.ReadAllText("tsconfig1.json"); dynamic jsonObj = JsonConvert.DeserializeObject(json); dShutterValue = jsonObj["exposureTime"]; m_txt_Shutter.Text = dShutterValue.ToString("F2"); m_listCCamerInfo[m_nOperateID].m_objIGXFeatureControl.GetFloatFeature("ExposureTime").SetValue(dShutterValue); m_txt_Shutter.Clear(); try { if (!m_listCCamerInfo[m_nOperateID].m_bIsOpen) { return; } strValue = m_listCCamerInfo[m_nOperateID].m_objIGXFeatureControl.GetFloatFeature("ExposureTime").GetValue().ToString(); //浮点转字符串 try { dShutterValue = Convert.ToDouble(m_txt_Shutter.Text);//把文本框的值换成double,给dShutterValue } catch (Exception) { MessageBox.Show("请输入正确的曝光值"); m_txt_Shutter.Text = strValue; return; }c#窗体的文本框被json文件赋值后无法继续接收键盘输入的值,怎样利用m_txt_Shutter.Clear();语句,使文本框被赋值后可以继续接受键盘输入的值
To allow the text box to continue accepting keyboard input after being assigned a value from the JSON file, you can remove the `m_txt_Shutter.Clear()` statement. This statement clears the current text in the text, which is why it appears that the text box cannot receive keyboard input after being assigned a value.
Here is the updated code:
```csharp
string json = File.ReadAllText("tsconfig1.json");
dynamic jsonObj = JsonConvert.DeserializeObject(json);
dShutterValue = jsonObj["exposureTime"];
m_txt_Shutter.Text = dShutterValue.ToString("F2");
m_listCCamerInfo[m_nOperateID].m_objIGXFeatureControl.GetFloatFeature("ExposureTime").SetValue(dShutterValue);
try
{
if (!m_listCCamerInfo[m_nOperateID].m_bIsOpen)
{
return;
}
strValue = m_listCCamerInfo[m_nOperateID].m_objIGXFeatureControl.GetFloatFeature("ExposureTime").GetValue().ToString();
//浮点转字符串
try
{
dShutterValue = Convert.ToDouble(m_txt_Shutter.Text); //把文本框的值换成double,给dShutterValue
}
catch (Exception)
{
MessageBox.Show("请输入正确的曝光值");
m_txt_Shutter.Text = strValue;
return;
}
}
catch (Exception ex)
{
// Handle any other exceptions here
// Log the exception or display an error message
}
```
By removing the `m_txt_Shutter.Clear()` statement, the text box will retain the value assigned from the JSON file and will be able to receive keyboard input.
C# 用Socket写一个点对点通信,要求客户端既是服务端又是服务端,界面上有连接按钮,断开按钮,发送按钮,两个RichTextBox控件,
以下是基于C#的Socket编写点对点通信的代码,客户端既是服务端又是服务端,具有连接、断开、发送等功能,界面上有连接按钮、断开按钮、发送按钮和两个RichTextBox控件:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading;
using System.Windows.Forms;
namespace P2PCommunicate
{
public partial class Form1 : Form
{
private Socket _serverSocket; // 服务端Socket
private Socket _clientSocket; // 客户端Socket
private Thread _serverThread; // 服务端线程
private Thread _clientThread; // 客户端线程
private bool _isServerStarted; // 服务端是否已启动
private bool _isClientStarted; // 客户端是否已启动
private string _serverIp = "127.0.0.1"; // 服务端IP地址
private int _serverPort = 8888; // 服务端端口号
private string _clientIp = "127.0.0.1"; // 客户端IP地址
private int _clientPort = 8889; // 客户端端口号
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
UpdateUI(true);
}
private void btnConnect_Click(object sender, EventArgs e)
{
if (!_isClientStarted)
{
_clientThread = new Thread(ClientThreadFunc);
_clientThread.Start();
}
else
{
MessageBox.Show("客户端已经连接!", "提示");
}
}
private void btnDisconnect_Click(object sender, EventArgs e)
{
if (_isClientStarted)
{
_clientSocket.Close();
_clientThread.Abort();
_isClientStarted = false;
UpdateUI(false);
}
else
{
MessageBox.Show("客户端未连接!", "提示");
}
}
private void btnSend_Click(object sender, EventArgs e)
{
if (_isClientStarted)
{
SendMessage(txtSend.Text);
txtSend.Clear();
}
else
{
MessageBox.Show("客户端未连接!", "提示");
}
}
private void ServerThreadFunc()
{
try
{
_serverSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
_serverSocket.Bind(new IPEndPoint(IPAddress.Parse(_serverIp), _serverPort));
_serverSocket.Listen(10);
while (true)
{
Socket client = _serverSocket.Accept();
Thread receiveThread = new Thread(ReceiveThreadFunc);
receiveThread.Start(client);
}
}
catch (Exception ex)
{
MessageBox.Show("服务端异常:" + ex.Message, "错误");
}
}
private void ClientThreadFunc()
{
try
{
_clientSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
_clientSocket.Connect(new IPEndPoint(IPAddress.Parse(_clientIp), _clientPort));
Thread receiveThread = new Thread(ReceiveThreadFunc);
receiveThread.Start(_clientSocket);
_isClientStarted = true;
UpdateUI(true);
}
catch (Exception ex)
{
MessageBox.Show("客户端异常:" + ex.Message, "错误");
}
}
private void ReceiveThreadFunc(object obj)
{
Socket socket = obj as Socket;
byte[] buffer = new byte[1024];
while (true)
{
int length = socket.Receive(buffer);
string message = Encoding.UTF8.GetString(buffer, 0, length);
AppendText(txtReceive, string.Format("[{0:HH:mm:ss}] {1}", DateTime.Now, message));
}
}
private void SendMessage(string message)
{
byte[] buffer = Encoding.UTF8.GetBytes(message);
_clientSocket.Send(buffer);
AppendText(txtReceive, string.Format("[{0:HH:mm:ss}] {1}", DateTime.Now, message));
}
private void AppendText(RichTextBox box, string text)
{
if (box.InvokeRequired)
{
box.Invoke(new Action<RichTextBox, string>(AppendText), box, text);
}
else
{
box.AppendText(text + Environment.NewLine);
}
}
private void UpdateUI(bool isConnected)
{
btnConnect.Enabled = !isConnected;
btnDisconnect.Enabled = isConnected;
btnSend.Enabled = isConnected;
txtSend.Enabled = isConnected;
txtReceive.Enabled = isConnected;
}
}
}
```
在窗体设计中,需要添加一个连接按钮(btnConnect)、一个断开按钮(btnDisconnect)、一个发送按钮(btnSend)、一个发送文本框(txtSend)、一个接收文本框(txtReceive)和两个标签(lblServer、lblClient)。
注意:该程序中客户端和服务端使用的是不同的端口号,可以根据实际需要进行修改。
阅读全文
相关推荐
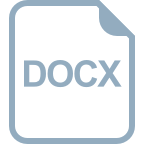
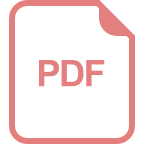
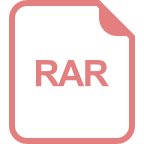
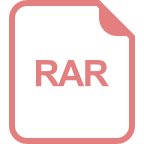
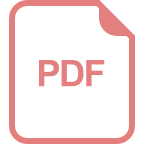
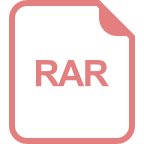
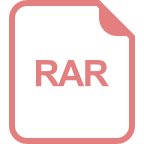
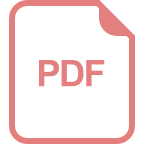
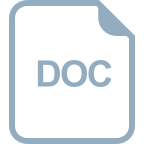
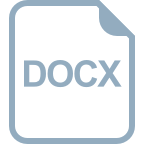
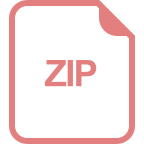
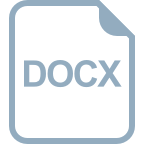
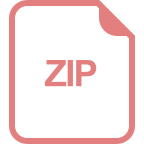