帮我用C++写一下题目:密码要求:1.长度超过8位;2.包括大小写字母、数字、其他符号,以上四种至少三种;3.不能有长度大于2的包括公共元素的子串重复(其他符号不含空格或换行);数据范围:输入的字符串长度满足1<=n<=100;输入描述:一串字符串;输出描述:如果符合要求输出:OK,否则输出NG
时间: 2024-04-14 20:28:09 浏览: 175
以下是用 C++ 编写的解答:
```cpp
#include <iostream>
#include <string>
#include <unordered_set>
bool checkPassword(const std::string& password) {
// 检查长度
if (password.length() <= 8) {
return false;
}
// 统计包含的字符种类
int categoryCount = 0;
bool hasLower = false;
bool hasUpper = false;
bool hasDigit = false;
bool hasSymbol = false;
for (char c : password) {
if (islower(c)) {
hasLower = true;
} else if (isupper(c)) {
hasUpper = true;
} else if (isdigit(c)) {
hasDigit = true;
} else {
hasSymbol = true;
}
}
if (hasLower) categoryCount++;
if (hasUpper) categoryCount++;
if (hasDigit) categoryCount++;
if (hasSymbol) categoryCount++;
// 检查字符种类是否符合要求
if (categoryCount < 3) {
return false;
}
// 检查重复子串
std::unordered_set<std::string> substrings;
for (int i = 0; i < password.length() - 2; i++) {
for (int j = i + 3; j <= password.length(); j++) {
std::string substring = password.substr(i, j - i);
if (substrings.count(substring)) {
return false;
}
substrings.insert(substring);
}
}
return true;
}
int main() {
std::string password;
std::cin >> password;
if (checkPassword(password)) {
std::cout << "OK" << std::endl;
} else {
std::cout << "NG" << std::endl;
}
return 0;
}
```
这段代码定义了一个 `checkPassword` 函数来检查密码是否符合要求。在 `main` 函数中,用户从标准输入中输入一个密码,并通过调用 `checkPassword` 函数来判断密码是否满足要求。如果满足要求,则输出 "OK",否则输出 "NG"。
`checkPassword` 函数首先检查密码的长度是否满足要求。然后,通过遍历密码中的每个字符,统计包含的字符种类,并计算字符种类的数量。接下来,使用哈希集合来存储所有的子串,并检查是否有重复的子串。如果满足所有要求,则返回 `true`,否则返回 `false`。
请注意,这只是一个简单的实现,并不能涵盖所有可能的情况。在实际应用中,可能需要根据具体要求进行进一步的优化和改进。
阅读全文
相关推荐
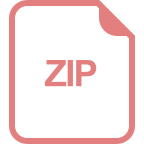

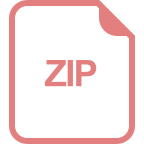
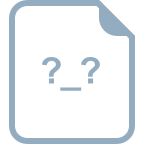
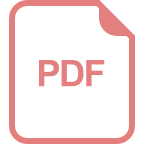
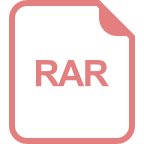
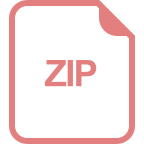
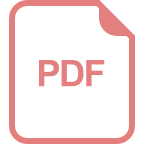
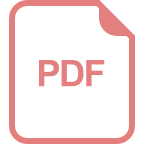
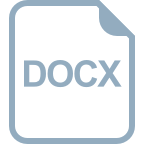
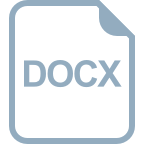
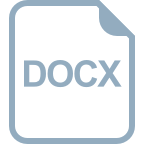
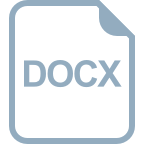
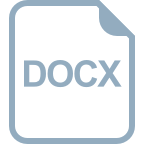
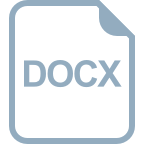
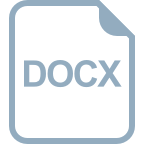
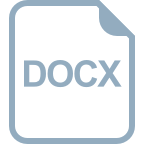