根据实体类 自动生成查询的linq
时间: 2024-09-20 22:15:48 浏览: 34
在.NET Core中,使用Entity Framework (EF) 和 LINQ(Language Integrated Query)可以帮助我们方便地生成针对数据库操作的查询,即使没有显式编写SQL语句。当你有一个实体类(比如`Product`),EF会自动映射这个类到数据库表上。以下是如何使用EF和LINQ进行基于实体类的查询:
```csharp
using System.Linq;
using Microsoft.EntityFrameworkCore;
// 假设你有一个名为`ProductContext`的DbContext类,继承自DbContext
public class ProductContext : DbContext
{
public DbSet<Product> Products { get; set; } // 假设Product是你的实体类
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseSqlServer("your_connection_string_here"); // 配置数据库连接
}
}
// 在控制器或服务中,你可以这样生成查询
public class ProductService
{
private readonly ProductContext _context;
public ProductService(ProductContext context)
{
_context = context;
}
// 查询所有产品
public List<Product> GetAllProducts()
{
return _context.Products.ToList(); // 使用ToLis()获取所有的实体
}
// 按名称搜索产品
public List<Product> SearchProducts(string searchTerm)
{
return _context.Products.Where(p => p.Name.Contains(searchTerm)).ToList();
}
// 分页查询
public async Task<List<Product>> GetProducts(int pageNumber, int pageSize)
{
int skip = (pageNumber - 1) * pageSize;
return await _context.Products.OrderBy(p => p.Name).Skip(skip).Take(pageSize).ToListAsync();
}
}
```
在这个例子中,`Where`, `OrderBy`, `Skip`, 和 `Take` 方法都是LINQ扩展方法,它们会生成动态的SQL查询并发送到数据库。
阅读全文
相关推荐
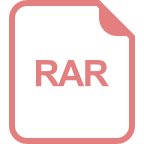
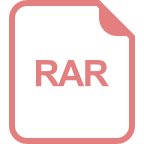
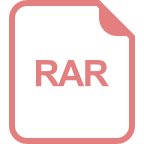
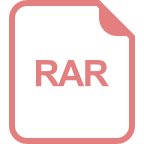
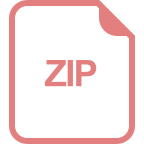
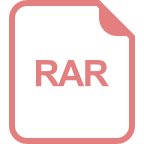
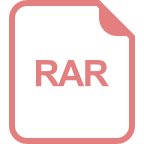
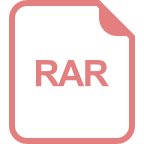
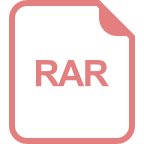
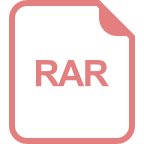
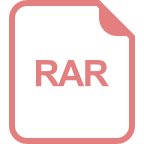
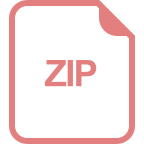
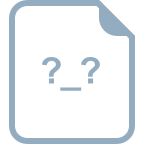
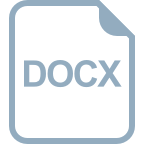
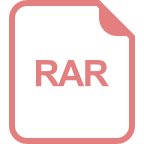
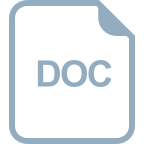
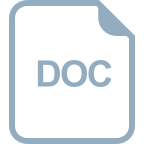
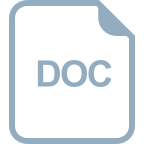
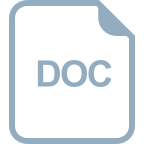
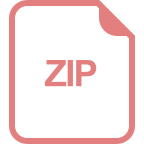