qt 线程进行数据交互
时间: 2023-11-30 22:03:52 浏览: 46
在Qt中,线程间的数据交互可以通过信号和槽机制实现。具体步骤如下:
1. 定义一个继承自QObject的类,在这个类中定义需要在不同线程间交互的信号和槽函数。
2. 在主程序中创建线程对象,并将这个QObject子类的对象移动到这个线程中,使得这个对象可以在线程中运行。
3. 在线程中连接信号和槽函数,以便在需要时进行数据交互。
下面是一个简单的示例代码,演示了如何在两个线程之间进行数据交互:
```cpp
// MyThread.h
#include <QObject>
#include <QThread>
class MyThread : public QThread
{
Q_OBJECT
public:
MyThread(QObject *parent = 0);
void setMessage(const QString &message);
void stop();
signals:
void messageChanged(const QString &message);
protected:
void run() override;
private:
QString m_message;
volatile bool m_stopped;
};
// MyThread.cpp
#include "MyThread.h"
MyThread::MyThread(QObject *parent)
: QThread(parent), m_stopped(false)
{
}
void MyThread::setMessage(const QString &message)
{
m_message = message;
}
void MyThread::stop()
{
m_stopped = true;
}
void MyThread::run()
{
while (!m_stopped) {
// do some work
// ...
// emit a signal to indicate that the message has changed
emit messageChanged(m_message);
// sleep for a bit to simulate doing some work
msleep(1000);
}
}
// MainWindow.h
#include <QMainWindow>
#include "MyThread.h"
namespace Ui {
class MainWindow;
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
private slots:
void onMessageChanged(const QString &message);
private:
Ui::MainWindow *ui;
MyThread *m_thread;
};
// MainWindow.cpp
#include "MainWindow.h"
#include "ui_MainWindow.h"
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow),
m_thread(new MyThread(this))
{
ui->setupUi(this);
// connect the messageChanged signal from the thread to the onMessageChanged slot in the main thread
connect(m_thread, &MyThread::messageChanged, this, &MainWindow::onMessageChanged);
// start the thread
m_thread->start();
}
MainWindow::~MainWindow()
{
delete ui;
m_thread->stop();
m_thread->wait();
delete m_thread;
}
void MainWindow::onMessageChanged(const QString &message)
{
// update the UI with the new message
ui->label->setText(message);
}
void MainWindow::on_pushButton_clicked()
{
// set a new message in the thread
QString message = ui->lineEdit->text();
m_thread->setMessage(message);
}
```
在这个例子中,我们创建了一个MyThread类,继承自QThread。这个类中有一个setMessage函数,可以设置一个新的消息,在run函数中不断发送messageChanged信号。在主程序中,我们创建了一个MainWindow类,将MyThread对象移动到这个线程中,并连接了messageChanged信号和onMessageChanged槽函数。当点击PushButton时,我们调用了MyThread的setMessage函数,设置了一个新的消息。这个消息会在MyThread中发送messageChanged信号,触发onMessageChanged槽函数,在主程序中更新UI。
相关推荐
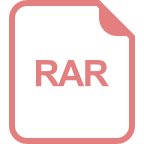














