javaweb数据库登陆操作
时间: 2023-08-07 17:09:01 浏览: 92
在JavaWeb中进行数据库登陆操作,需要先建立数据库连接。一般使用JDBC技术来实现数据库连接。
以下是一个简单的JavaWeb登陆操作的示例:
1. 导入JDBC驱动
```java
Class.forName("com.mysql.jdbc.Driver");
```
2. 建立数据库连接
```java
String url="jdbc:mysql://localhost:3306/test";
String user="root";
String password="123456";
Connection conn=DriverManager.getConnection(url,user,password);
```
3. 编写SQL语句
```java
String sql="select * from user where username=? and password=?";
```
4. 预处理SQL语句
```java
PreparedStatement pstmt=conn.prepareStatement(sql);
pstmt.setString(1,username);
pstmt.setString(2,password);
```
5. 执行SQL语句
```java
ResultSet rs=pstmt.executeQuery();
```
6. 判断是否登陆成功
```java
if(rs.next()){
//登陆成功
}else{
//登陆失败
}
```
完整的JavaWeb登陆操作代码如下:
```java
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username=request.getParameter("username");
String password=request.getParameter("password");
try {
Class.forName("com.mysql.jdbc.Driver");
String url="jdbc:mysql://localhost:3306/test";
String user="root";
String password="123456";
Connection conn=DriverManager.getConnection(url,user,password);
String sql="select * from user where username=? and password=?";
PreparedStatement pstmt=conn.prepareStatement(sql);
pstmt.setString(1,username);
pstmt.setString(2,password);
ResultSet rs=pstmt.executeQuery();
if(rs.next()){
//登陆成功
response.sendRedirect("success.jsp");
}else{
//登陆失败
response.sendRedirect("failure.jsp");
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
阅读全文
相关推荐
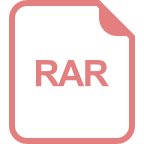
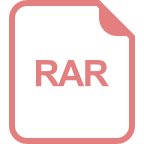
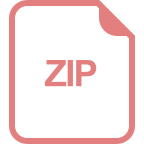















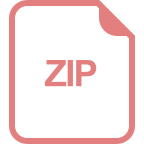