利用python和OpenCV实现车牌识别项目,并给出完整的算法讲解和代码。
时间: 2023-06-24 15:03:54 浏览: 120
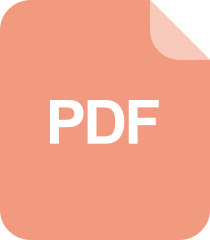
python+OpenCV实现车牌号码识别
车牌识别是计算机视觉中的一个重要应用。本文将介绍如何利用Python和OpenCV实现车牌识别项目,并给出完整的算法讲解和代码。
1. 算法流程
车牌识别的算法流程如下:
1. 读入图像
2. 预处理(灰度化、滤波、二值化)
3. 车牌定位
4. 车牌字符分割
5. 字符识别
下面我们将详细介绍每个步骤的实现方法。
2. 代码实现
以下是完整的代码实现:
```python
import cv2
import numpy as np
import pytesseract
# 读入图像
img = cv2.imread('car.jpg')
# 灰度化
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 高斯滤波
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# Sobel算子边缘检测
sobel = cv2.Sobel(blur, cv2.CV_8U, 1, 0, ksize=3)
# 二值化
ret, binary = cv2.threshold(sobel, 0, 255, cv2.THRESH_OTSU + cv2.THRESH_BINARY)
# 膨胀
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (17, 5))
binary = cv2.dilate(binary, kernel)
# 查找轮廓
contours, hierarchy = cv2.findContours(binary, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
# 筛选符合条件的轮廓
rects = []
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
if w > h and 5 < w < 17 * h and cv2.contourArea(contour) > 100:
rect = (x, y, w, h)
rects.append(rect)
# 画出符合条件的轮廓
for rect in rects:
cv2.rectangle(img, (rect[0], rect[1]), (rect[0] + rect[2], rect[1] + rect[3]), (0, 0, 255), 2)
# 车牌字符分割
for i, rect in enumerate(rects):
x, y, w, h = rect
roi = binary[y:y + h, x:x + w]
# 统计每一列的像素值
col_sum = np.sum(roi, axis=0)
# 计算字符边界
left = 0
right = len(col_sum) - 1
while left < len(col_sum) and col_sum[left] == 0:
left += 1
while right >= 0 and col_sum[right] == 0:
right -= 1
roi = roi[:, left:right + 1]
# 调整字符大小
scale = 40.0 / roi.shape[1]
roi = cv2.resize(roi, (0, 0), fx=scale, fy=scale)
# 识别字符
text = pytesseract.image_to_string(roi, lang='eng', config='--psm 10')
cv2.putText(img, text, (rect[0], rect[1]), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
# 显示结果
cv2.imshow('img', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
3. 算法讲解
下面我们将详细介绍每个步骤的实现方法。
3.1 读入图像
利用OpenCV的imread函数读入图像。
```python
img = cv2.imread('car.jpg')
```
3.2 预处理
车牌识别需要对图像进行预处理。首先将彩色图像转换为灰度图像,然后进行高斯滤波,最后进行Sobel算子边缘检测。
```python
# 灰度化
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 高斯滤波
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# Sobel算子边缘检测
sobel = cv2.Sobel(blur, cv2.CV_8U, 1, 0, ksize=3)
```
3.3 二值化
将边缘图像进行二值化处理。
```python
ret, binary = cv2.threshold(sobel, 0, 255, cv2.THRESH_OTSU + cv2.THRESH_BINARY)
```
3.4 膨胀
对二值化后的图像进行膨胀操作,使车牌区域更加明显。
```python
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (17, 5))
binary = cv2.dilate(binary, kernel)
```
3.5 查找轮廓
使用OpenCV的findContours函数查找轮廓。
```python
contours, hierarchy = cv2.findContours(binary, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
```
3.6 筛选符合条件的轮廓
使用筛选条件对轮廓进行筛选,得到车牌区域的轮廓。
```python
rects = []
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
if w > h and 5 < w < 17 * h and cv2.contourArea(contour) > 100:
rect = (x, y, w, h)
rects.append(rect)
```
3.7 画出符合条件的轮廓
将符合条件的轮廓画出来,观察是否正确。
```python
for rect in rects:
cv2.rectangle(img, (rect[0], rect[1]), (rect[0] + rect[2], rect[1] + rect[3]), (0, 0, 255), 2)
```
3.8 车牌字符分割
在得到车牌区域的轮廓之后,需要对车牌字符进行分割。首先将车牌区域进行二值化处理,然后统计每一列的像素值,计算出字符的左右边界,并进行调整大小。最后使用Tesseract OCR进行字符识别。
```python
for i, rect in enumerate(rects):
x, y, w, h = rect
roi = binary[y:y + h, x:x + w]
# 统计每一列的像素值
col_sum = np.sum(roi, axis=0)
# 计算字符边界
left = 0
right = len(col_sum) - 1
while left < len(col_sum) and col_sum[left] == 0:
left += 1
while right >= 0 and col_sum[right] == 0:
right -= 1
roi = roi[:, left:right + 1]
# 调整字符大小
scale = 40.0 / roi.shape[1]
roi = cv2.resize(roi, (0, 0), fx=scale, fy=scale)
# 识别字符
text = pytesseract.image_to_string(roi, lang='eng', config='--psm 10')
cv2.putText(img, text, (rect[0], rect[1]), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
```
4. 总结
本文介绍了利用Python和OpenCV实现车牌识别项目的算法流程和代码实现方法。通过对图像进行预处理、车牌定位、字符分割和字符识别等步骤,可以实现对车牌的准确识别。
阅读全文
相关推荐
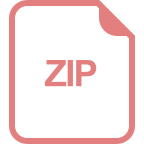
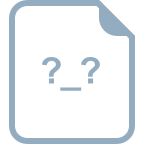
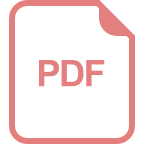
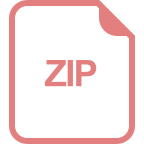
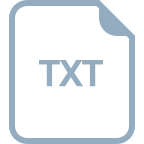
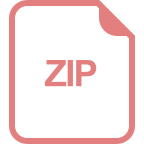
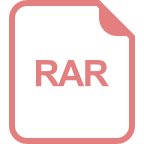
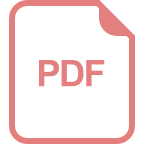
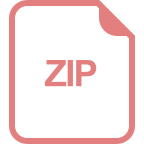
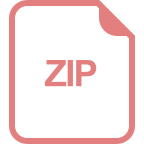
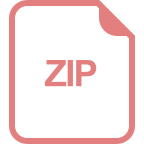