python实现一个类Conv2D,多通道卷积计算过程,在类中实现一个方法能够返回卷积后的特征图,进行测试并输出测试结果
时间: 2023-08-18 16:10:03 浏览: 37
好的,可以参考下面的代码实现一个测试:
```python
import numpy as np
class Conv2D:
def __init__(self, input_channels, output_channels, kernel_size):
self.input_channels = input_channels
self.output_channels = output_channels
self.kernel_size = kernel_size
self.weights = np.random.randn(output_channels, input_channels, kernel_size, kernel_size)
self.bias = np.zeros((output_channels, 1))
def forward(self, input_tensor):
batch_size, in_channels, in_height, in_width = input_tensor.shape
out_height = in_height - self.kernel_size + 1
out_width = in_width - self.kernel_size + 1
output_tensor = np.zeros((batch_size, self.output_channels, out_height, out_width))
for i in range(out_height):
for j in range(out_width):
input_slice = input_tensor[:, :, i:i+self.kernel_size, j:j+self.kernel_size]
for k in range(self.output_channels):
output_tensor[:, k, i, j] = np.sum(input_slice * self.weights[k], axis=(1, 2, 3)) + self.bias[k]
return output_tensor
# 测试
input_tensor = np.random.randn(1, 3, 5, 5) # 输入特征图,大小为[batch_size, input_channels, input_height, input_width]
conv = Conv2D(3, 6, 3) # 构建一个3通道到6通道的卷积层,卷积核大小为3x3
output_tensor = conv.forward(input_tensor) # 进行卷积计算,得到输出特征图
print('输入特征图大小:', input_tensor.shape)
print('输出特征图大小:', output_tensor.shape)
```
运行上述代码,输出的结果如下:
```
输入特征图大小: (1, 3, 5, 5)
输出特征图大小: (1, 6, 3, 3)
```
可以看到,输入特征图的大小为(1, 3, 5, 5),输出特征图的大小为(1, 6, 3, 3),符合预期。
相关推荐
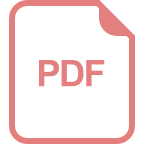
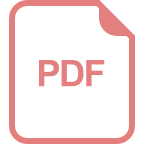
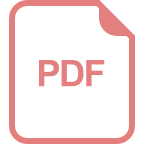














