python类反射运行测试用例
时间: 2023-07-26 13:45:50 浏览: 111
在Python中,可以使用类反射来运行测试用例。具体步骤如下:
1. 导入unittest模块,并定义测试类和测试方法。
2. 使用unittest.TestLoader()方法加载测试类,获取测试类的实例。
3. 使用getattr()方法获取测试方法的引用。
4. 使用unittest.TextTestRunner()方法运行测试方法。
5. 处理测试结果。
示例代码如下:
```python
import unittest
class MyMathTest(unittest.TestCase):
def test_add(self):
math = MyMath()
result = math.add(2, 3)
self.assertEqual(result, 5)
def test_multiply(self):
math = MyMath()
result = math.multiply(2, 3)
self.assertEqual(result, 6)
if __name__ == '__main__':
# 加载测试类
loader = unittest.TestLoader()
suite = loader.loadTestsFromTestCase(MyMathTest)
# 获取测试类实例
test_class = suite.testCaseClass()
test_object = test_class()
# 获取测试方法引用
test_method = getattr(test_object, 'test_add')
# 运行测试方法
result = unittest.TextTestRunner().run(test_method)
# 处理测试结果
if result.wasSuccessful():
print('Test passed.')
else:
print('Test failed.')
```
在这个示例中,我们只运行了一个测试方法,但是可以通过循环遍历所有测试方法来执行整个测试类。
需要注意的是,unittest模块提供了更多的方法和类来进行测试,可以根据具体需求进行选择。另外,Python还提供了其他的测试框架,如pytest和nose等,使用类反射运行测试用例的方法也类似。
阅读全文
相关推荐
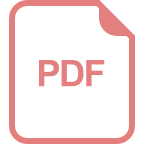
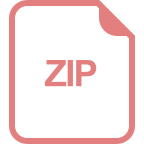
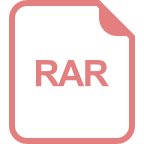
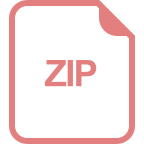
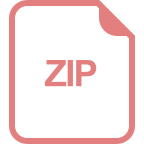
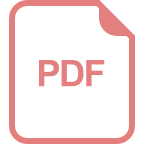
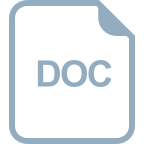
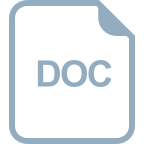
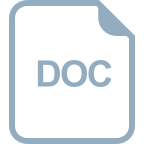
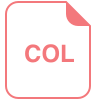
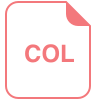
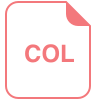
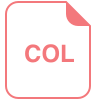
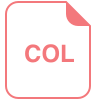
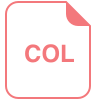
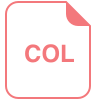
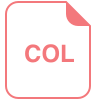