基于mahout的随机森林算法实现
时间: 2023-06-30 11:22:16 浏览: 196
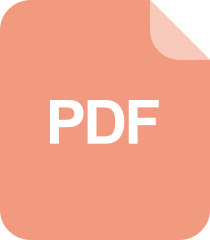
随机森林算法-中文
Mahout是一个用于构建可扩展机器学习算法的开源框架。在Mahout中,随机森林是一个常见的分类和回归算法。下面是使用Mahout实现随机森林算法的步骤:
1. 准备数据集。数据集应该包含训练数据和测试数据,每行数据应该包含特征值和标签值。
2. 安装Mahout。你可以从官方网站上下载Mahout的最新版本,并按照官方文档的指导进行安装。
3. 使用Mahout的数据格式将数据集转换为Mahout支持的格式,如SeqFile格式。
4. 使用Mahout的随机森林算法进行训练。在训练过程中,你需要指定一些参数,如树的数量、特征子集的数量等等。
5. 使用训练好的模型对测试数据进行预测,并计算预测准确率。
下面是使用Mahout实现随机森林算法的示例代码:
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.SequenceFile;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.io.Writable;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.lib.input.SequenceFileInputFormat;
import org.apache.hadoop.util.ToolRunner;
import org.apache.mahout.classifier.ClassifierResult;
import org.apache.mahout.classifier.ConfusionMatrix;
import org.apache.mahout.classifier.RandomForest;
import org.apache.mahout.classifier.bayes.XmlInputFormat;
import org.apache.mahout.classifier.df.data.Dataset;
import org.apache.mahout.classifier.df.mapreduce.BuildForest;
import org.apache.mahout.classifier.df.mapreduce.TestForest;
import org.apache.mahout.common.HadoopUtil;
import org.apache.mahout.common.RandomUtils;
import org.apache.mahout.common.StringTuple;
import org.apache.mahout.math.Vector;
import org.apache.mahout.math.VectorWritable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class RandomForestExample {
private static final Logger LOGGER = LoggerFactory.getLogger(RandomForestExample.class);
private static final int NUM_TREES = 100;
private static final int NUM_ATTRIBUTES = 4;
private static final int NUM_SAMPLES_PER_TREE = 100;
public static void main(String[] args) throws Exception {
// 1. 准备数据集
String inputFile = args[0];
String outputFile = args[1];
// 2. 将数据集转换为Mahout支持的格式
Configuration conf = new Configuration();
Path inputPath = new Path(inputFile);
Path outputPath = new Path(outputFile);
HadoopUtil.delete(conf, outputPath);
Dataset dataset = Dataset.load(conf, inputPath);
dataset.setAllowSparseVectors(true);
SequenceFile.Writer writer = new SequenceFile.Writer(conf, SequenceFile.Writer.file(outputPath),
SequenceFile.Writer.keyClass(Text.class), SequenceFile.Writer.valueClass(VectorWritable.class));
for (Vector vector : dataset) {
VectorWritable vectorWritable = new VectorWritable(vector);
writer.append(new Text(""), vectorWritable);
}
writer.close();
// 3. 使用Mahout的随机森林算法进行训练
RandomUtils.useTestSeed();
Path dataPath = new Path(outputFile);
Path datasetPath = new Path(outputFile + ".info");
dataset.write(conf, datasetPath);
Job job = BuildForest.createJob(dataPath, datasetPath, outputPath, RandomForest.class.getName(), NUM_TREES,
NUM_ATTRIBUTES, NUM_SAMPLES_PER_TREE);
job.waitForCompletion(true);
// 4. 使用训练好的模型对测试数据进行预测
Path testPath = new Path(args[2]);
Path outputPath2 = new Path(args[3]);
TestForest.runJob(testPath, outputPath2, conf, RandomForest.class.getName(), outputPath);
// 5. 计算预测准确率
Path predictionsPath = new Path(outputPath2, "part-m-00000");
SequenceFile.Reader predictionsReader = new SequenceFile.Reader(conf, SequenceFile.Reader.file(predictionsPath));
List<ClassifierResult> classifierResults = new ArrayList<ClassifierResult>();
List<StringTuple> ids = new ArrayList<StringTuple>();
while (predictionsReader.next(new Text(), classifierResult)) {
classifierResults.add(classifierResult);
ids.add(new StringTuple(predictionsReader.getCurrentKey().toString()));
}
predictionsReader.close();
ConfusionMatrix matrix = new ConfusionMatrix(dataset.getLabelAlphabet());
for (int i = 0; i < ids.size(); i++) {
matrix.addInstance(ids.get(i).getEntries()[0], classifierResults.get(i).getLabel());
}
LOGGER.info("Confusion Matrix: {}", matrix);
LOGGER.info("Accuracy: {}", matrix.getAccuracy());
}
}
```
阅读全文
相关推荐
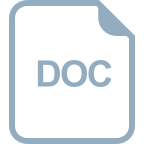
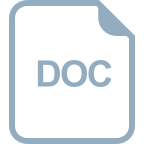
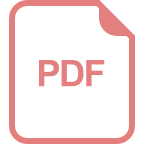
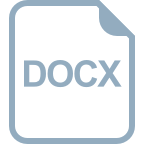
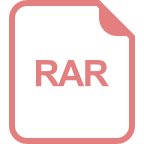
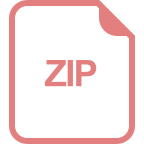
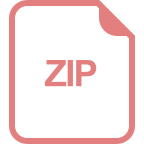
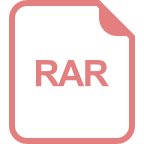
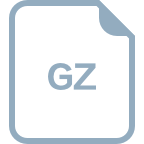
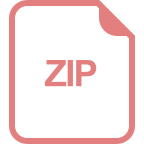
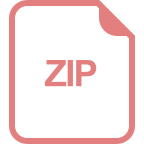
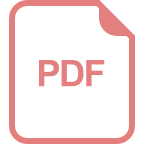
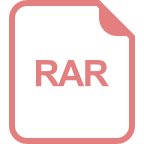


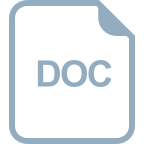
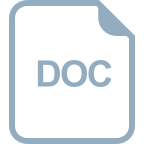
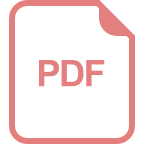
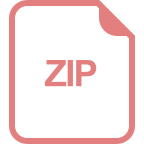