飞机大战依据项目需求,实现代码开发:①生成可操控的英雄飞机,能够实现基础移动和发射子弹。②生成自动运行的敌机,能够实现自动移动和自动发射子弹。③添加背景音乐和背景图片。④实现英雄飞机和敌机的碰撞检测,并触发销毁。
时间: 2024-11-11 22:23:13 浏览: 11
要实现这样一个"飞机大战"游戏,你需要使用Python的游戏开发库如pygame或 arcade,它们提供了丰富的图形渲染、音频处理和事件管理功能。以下是一个简化的步骤概述:
1. **安装依赖**:
首先确保已经安装了`pygame`库,如果没有,可以通过pip安装:
```
pip install pygame
```
2. **创建游戏窗口**:
使用pygame初始化一个新的窗口,设置背景音乐和背景图片:
```python
import pygame
# 初始化pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("飞机大战")
background_music = pygame.mixer.music.load('background_music.mp3')
pygame.mixer.music.play(-1) # -1表示无限循环
bg_surface = pygame.image.load('background.jpg').convert()
```
3. **定义英雄飞机类(PlayerPlane)**:
创建一个类,包含位置、速度等属性,以及移动和射击的方法:
```python
class PlayerPlane(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = pygame.Surface((50, 50)) # 设置飞机图像
self.rect = self.image.get_rect() # 获取矩形区域
self.pos = (50, 500) # 初始位置
self.speed = 5
def move(self, dx, dy):
self.rect.x += dx
self.rect.y += dy
def shoot(self):
bullet = Bullet(self.rect.centerx, self.rect.top)
all_sprites.add(bullet)
```
4. **定义敌机类(EnemyPlane)**:
类似于英雄飞机,但不需要玩家控制,可以随机生成并移动:
```python
class EnemyPlane(pygame.sprite.Sprite):
def __init__(self):
...
def update(self):
...
```
5. **创建精灵组(Sprite Groups)**:
使用`pygame.sprite.Group`来组织和更新所有飞机和子弹:
```python
all_sprites = pygame.sprite.Group()
player_plane = PlayerPlane()
all_sprites.add(player_plane)
enemies = pygame.sprite.Group()
for _ in range(10): # 创建10个敌机
enemy_plane = EnemyPlane()
enemies.add(enemy_plane)
all_sprites.add(enemy_plane)
```
6. **碰撞检测**:
让飞机类继承自`pygame.spritecollideany()`方法,用于检查是否与任何其他精灵发生碰撞:
```python
class CollidableSprite(pygame.sprite.Sprite):
def collide(self, other):
return pygame.sprite.collide_mask(self, other)
PlayerPlane = CollidableSprite
EnemyPlane = CollidableSprite
```
7. **主游戏循环**:
更新精灵组,绘制到屏幕,处理事件(如键盘输入),并在发生碰撞时销毁飞机:
```python
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
all_sprites.update()
collisions = pygame.sprite.groupcollide(all_sprites, all_sprites, False, True)
# 检查碰撞
if collisions:
for hit_list in collisions.values():
for sprite in hit_list:
if isinstance(sprite, PlayerPlane):
print("Player Plane Destroyed!")
else:
print(f"Enemy Plane {sprite} Destroyed!")
screen.blit(bg_surface, (0, 0))
all_sprites.draw(screen)
pygame.display.flip()
clock.tick(60) # 控制帧率
```
阅读全文
相关推荐
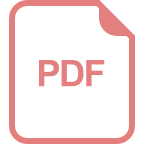
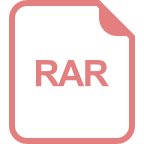
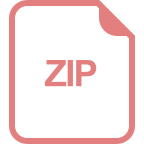
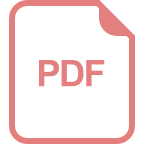
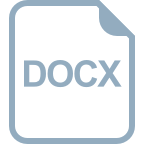
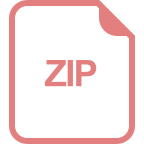
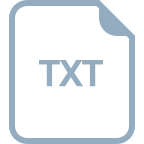
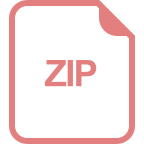
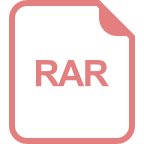
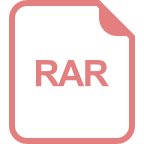
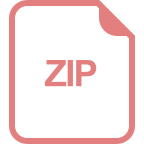
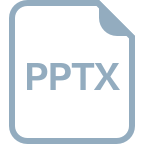
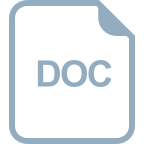
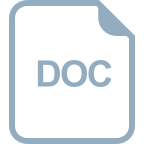
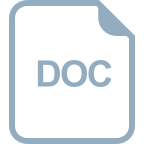
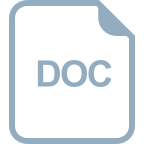
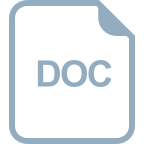


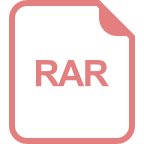