def decoder_block_resize(x, x_skip, filters, kernel_size, padding='same', dilation_rate=1): """ Decoder block used in expansive path of UNet. Unlike before, this block resizes the skip connections rather than cropping. """ # print(x.shape) # print(x_skip.shape[1:3]) x = tf.image.resize(x, x_skip.shape[1:3], method='nearest') x = layers.concatenate([x, x_skip], axis=3) x = conv2d_block(x, filters, kernel_size, padding, dilation_rate, batch_norm=True, activation='relu') x = conv2d_block(x, filters, kernel_size, padding, dilation_rate, batch_norm=True, activation='relu') x = conv2d_block(x, filters, kernel_size, padding, dilation_rate, batch_norm=True, activation='relu')
时间: 2024-01-22 07:02:47 浏览: 64
这是一个用于UNet中扩张路径的解码器块,与之前不同的是,该块重新调整跳跃连接的大小而不是裁剪。该函数的输入参数包括 x,x_skip,filters,kernel_size,padding 和 dilation_rate 等。其中 x 和 x_skip 分别代表解码器输入和跳跃连接输入,filters 代表卷积核数量,kernel_size 代表卷积核大小,padding 代表填充方式,dilation_rate 代表膨胀率。函数的核心操作包括对 x 进行 resize 操作,将 x 和 x_skip 进行 concatenate 操作,以及三次卷积操作。
相关问题
inputs = tf.keras.Input((height, width, 1)) x = inputs # Multi-Wiener deconvol utions x = WienerDeconvolution(initial_psf, initial_K)(x) skips = [] # Contracting path for c in encoding_cs: x, x_skip = encoder_block(x, c, kernel_size=3, padding='same', dilation_rate=1, pooling='average') skips.append(x_skip) skips = list(reversed(skips)) # Center x = conv2d_block(x, center_cs, kernel_size=3, padding='same') # Expansive path for i, c in enumerate(decoding_cs): if skip_connections[i]: x = decoder_block_resize(x, skips[i], c, kernel_size=3, padding='same', dilation_rate=1) else: x = decoder_block(x, None, c, kernel_size=3, padding='same', dilation_rate=1) # Classify x = layers.Conv2D(filters=1, kernel_size=1, use_bias=True, activation='relu')(x) outputs = tf.squeeze(x, axis=3) model = tf.keras.Model(inputs=[inputs], outputs=[outputs])
这段代码是一个基于深度学习的图像去模糊模型,使用了U-Net的结构。代码中包含了两个子模块:编码器和解码器。编码器通过多个卷积层将输入图像压缩为特征图,其中的池化操作可以缩小特征图的尺寸。在编码器的每一层中,都会保存一个跳跃连接,将该层的特征图保存下来,以便在解码器中恢复图像细节时使用。解码器则将特征图进行上采样,逐渐恢复图像细节,每一层都会将对应编码器层的跳跃连接特征图进行拼接,以恢复更多的细节。最后,使用一个卷积层将输出压缩为一维,得到最终的去模糊结果。
def DeepLabV3Plus(input_shape = (256,256,3), num_classes = 3): inputs = Input(shape=input_shape) # Encoder encoder_output, skip_1, skip_2, skip_3 = encoder(inputs) # ASPP (Atrous Spatial Pyramid Pooling) x = conv_block(encoder_output, 256, kernel_size=1) x = conv_block(x, 256, kernel_size=3, strides=1, dilation_rate=6) x = conv_block(x, 256, kernel_size=3, strides=1, dilation_rate=12) x = conv_block(x, 256, kernel_size=3, strides=1, dilation_rate=18) x = Conv2D(256, 1)(x) x = BatchNormalization()(x) # Decoder x = decoder(x, skip_1, skip_2, skip_3) # Output outputs = Conv2D(num_classes, 1, activation='softmax')(x) model = Model(inputs=inputs, outputs=outputs) model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy']) return model
这是一个使用DeepLabV3Plus架构的图像分割模型。它由以下几个部分组成:
1. Encoder:从输入图像中提取特征,并生成skip连接以供解码器使用。
2. ASPP(空洞空间金字塔池化):通过使用不同的扩张率(dilation rate)来捕捉不同尺度的上下文信息。
3. Decoder:使用skip连接和上采样操作将特征重新恢复到原始尺寸。
4. 输出层:使用1x1卷积将特征图映射到目标类别数量,并使用softmax激活函数进行分类。
该模型使用adam优化器,并使用分类交叉熵作为损失函数进行训练。你可以根据自己的数据集和要解决的问题调整输入形状和类别数量。
阅读全文
相关推荐












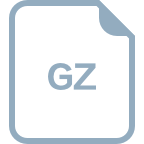



