在idea中用ORACLE请用三种LOOP循环实现12! 2、请自定义异常并处理
时间: 2024-12-24 12:31:58 浏览: 4
在IntelliJ IDEA中使用Oracle数据库,并通过三种不同的循环结构(for,while,do-while)来计算阶乘12!,我们首先需要创建连接,然后编写一个递归函数来计算阶乘。以下是示例代码:
1. For循环实现:
```java
try {
// 连接Oracle数据库(这里假设已配置好环境)
OracleConnection conn = ...;
// 定义阶乘计算函数
long factorial(int n) {
if (n == 0 || n == 1) return 1; // 阶乘的基本情况
else return n * factorial(n - 1);
}
// 使用for循环计算12!
int n = 12;
long result = factorial(n);
// 输出结果
System.out.println("12! 的阶乘结果是:" + result);
} catch (SQLException e) {
// 抛出并捕获自定义异常
throw new CustomDatabaseException("Error calculating factorial", e);
} finally {
// 关闭数据库连接
try {
conn.close();
} catch (SQLException e) {
// 如果关闭失败,记录错误
log.error("Failed to close database connection", e);
}
}
// 自定义异常类
class CustomDatabaseException extends SQLException {
public CustomDatabaseException(String message, Throwable cause) {
super(message, cause);
}
}
```
2. While循环实现:
```java
CustomDatabaseException customEx = null;
try {
// 同样连接Oracle数据库...
int n = 12;
int fact = 1;
while (n > 0) {
fact *= n;
n--;
}
// 计算完成,打印结果
System.out.println("12! 的阶乘结果是:" + fact);
} catch (SQLException e) {
// 捕获异常
customEx = new CustomDatabaseException("While loop error", e);
}
if (customEx != null) {
throw customEx;
}
// 同上,包含自定义异常类和finally块
```
3. Do-while循环实现:
```java
CustomDatabaseException customEx = null;
try {
// 连接Oracle数据库...
int n = 12, fact = 1;
do {
fact *= n;
n--;
} while (n > 0);
// 输出结果
System.out.println("12! 的阶乘结果是:" + fact);
} catch (SQLException e) {
// 捕获异常
customEx = new CustomDatabaseException("Do-while loop error", e);
}
if (customEx != null) {
throw customEx;
}
// 同样,包含自定义异常类和finally块
```
阅读全文
相关推荐
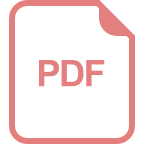
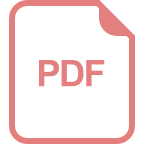
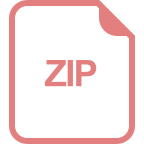















