设计一个完整的函数设计一个类CRectangle,除了有相关的数据成员外,该类还要求包含以下成员函数:矩形从一个位置移动到另一个位置的Move()函数;改变矩形大小的Size()函数;返回矩形左上角的坐标值的Where()函数;计算矩形面积的Area()函数。 点击复制后,将打开C知道体验页 好
时间: 2023-05-20 13:07:37 浏览: 86
的,以下是CRectangle类的完整代码:
class CRectangle {
public:
//构造函数
CRectangle(int x, int y, int w, int h) {
m_x = x;
m_y = y;
m_width = w;
m_height = h;
}
//移动函数
void Move(int x, int y) {
m_x += x;
m_y += y;
}
//尺寸函数
void Size(int w, int h) {
m_width = w;
m_height = h;
}
//位置函数
std::pair<int, int> Where() {
return std::make_pair(m_x, m_y);
}
//面积函数
int Area() {
return m_width * m_height;
}
private:
int m_x; //左上角坐标x
int m_y; //左上角坐标y
int m_width; //矩形宽度
int m_height; //矩形高度
};
相关问题
c++设计完整函数,1. 程序设计题:设计一个类CRectangle,除了有相关的数据成员外,该类还要求包含以下成员函数:矩形从一个位置移动到另一个位置的Move()函数;改变矩形大小的Size()函数;返回矩形左上角的坐标值的Where()函数;计算矩形面积的Area()函数。
以下是CRectangle类的完整代码:
class CRectangle {
private:
int m_left;
int m_top;
int m_right;
int m_bottom;
public:
CRectangle(int left, int top, int right, int bottom) {
m_left = left;
m_top = top;
m_right = right;
m_bottom = bottom;
}
void Move(int x, int y) {
m_left += x;
m_top += y;
m_right += x;
m_bottom += y;
}
void Size(int width, int height) {
m_right = m_left + width;
m_bottom = m_top + height;
}
int* Where() {
int* pos = new int[2];
pos[0] = m_left;
pos[1] = m_top;
return pos;
}
int Area() {
return (m_right - m_left) * (m_bottom - m_top);
}
};
使用示例:
CRectangle rect(0, 0, 10, 10);
rect.Move(5, 5);
rect.Size(20, 20);
int* pos = rect.Where();
int area = rect.Area();
注意:在使用Where()函数返回坐标值时,需要手动释放返回的指针,以避免内存泄漏。
1. 程序设计题:设计一个类CRectangle,除了有相关的数据成员外,该类还要求包含以下成员函数:矩形从一个位置移动到另一个位置的Move()函数;改变矩形大小的Size()函数;返回矩形左上角的坐标值的Where()函数;计算矩形面积的Area()函数。
好的,下面是我的回答。这是一个非常基础的面向对象编程题。您可以按照以下方式设计CRectangle类:
```
class CRectangle {
private:
int m_nLeft; // 矩形左上角x坐标
int m_nTop; // 矩形左上角y坐标
int m_nWidth; // 矩形宽度
int m_nHeight; // 矩形高度
public:
void Move(int nNewLeft, int nNewTop) {
m_nLeft = nNewLeft;
m_nTop = nNewTop;
}
void Size(int nNewWidth, int nNewHeight) {
m_nWidth = nNewWidth;
m_nHeight = nNewHeight;
}
void Where(int& nLeft, int& nTop) const {
nLeft = m_nLeft;
nTop = m_nTop;
}
int Area() const {
return m_nWidth * m_nHeight;
}
};
```
其中,Move()函数可以将矩形移动到指定位置,Size()函数可以改变矩形的大小,Where()函数可以获取矩形左上角的坐标值,Area()函数可以计算矩形的面积。
注意,在Where()函数中,由于需要返回两个值,我们采用了C++中的引用传参方式,这样可以实现在函数中同时返回多个值的效果。
这是一个简单的实现,您可以根据实际情况进行相应的修改和优化。
阅读全文
相关推荐
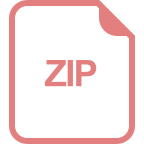
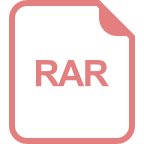












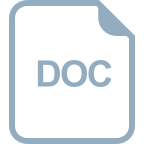
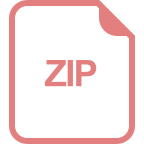