移动机器人路径规划Matlab
时间: 2023-06-25 12:07:55 浏览: 137
在Matlab中,可以使用Robotics System Toolbox来进行移动机器人路径规划,具体步骤如下:
1. 定义机器人模型:使用Robotics System Toolbox中的机器人模型函数来定义机器人的几何形状和运动学参数。
2. 创建地图:构建机器人将要在其中运动的环境地图,可以使用OccupancyGrid类来表示地图。
3. 配置路径规划器:选择合适的路径规划算法,例如A*,Dijkstra或RRT等,并配置规划器的参数。
4. 设置起点和终点:设置机器人的起点和终点,可以通过手动指定或者使用传感器获取。
5. 进行路径规划:使用规划器计算出机器人从起点到终点的最优路径。
6. 可视化结果:使用Matlab的图形化工具箱可视化路径规划结果,例如显示机器人在地图上的运动轨迹和避障结果等。
以上是移动机器人路径规划的大致流程,在实际使用中需要根据具体情况进行调整和优化。
相关问题
移动机器人路径规划Matlab代码
以下是一个简单的移动机器人路径规划的Matlab代码示例:
```matlab
%设定机器人起点和终点
start_point = [0,0];
end_point = [10,10];
%设定机器人的障碍物
obstacles = [4,4; 5,4; 6,4; 7,4; 8,4; 4,6; 5,6; 6,6; 7,6; 8,6;];
%设定地图边界
map_boundary = [0,0; 0,15; 15,15; 15,0;];
%生成地图并显示
figure(1)
hold on;
plot(start_point(1),start_point(2),'ro','MarkerFaceColor','r','MarkerSize',10);
plot(end_point(1),end_point(2),'go','MarkerFaceColor','g','MarkerSize',10);
plot(obstacles(:,1),obstacles(:,2),'ks','MarkerFaceColor','k','MarkerSize',10);
plot(map_boundary(:,1),map_boundary(:,2),'b-');
axis([0,15,0,15]);
grid on;
title('Robot Path Planning');
xlabel('X');
ylabel('Y');
%使用A*算法进行路径规划
path = A_star(start_point, end_point, obstacles, map_boundary);
%绘制路径
plot(path(:,1),path(:,2),'r-','LineWidth',2);
```
其中,`A_star`函数是一个实现A*算法的函数,可以根据具体情况自行编写。此代码示例中,机器人起点为`(0,0)`,终点为`(10,10)`,障碍物为`obstacles`,地图边界为`map_boundary`。在绘制地图时,起点、终点、障碍物分别用不同的颜色和形状表示。最后,使用A*算法计算路径,并在地图上绘制出来。
24邻域 a* 算法的移动机器人路径规划matlab代码
24邻域 A* 算法是一种常用于移动机器人路径规划的算法。下面是一个用 MATLAB 实现的示例代码:
```matlab
function path = a_star(start, goal, obstacle)
dx = [-1, 0, 1, -1, 1, -1, 0, 1, -1, 0, 1, -1, 1, -1, 0, 1, -1, 0, 1, -1, 0, 1, -1, 0, 1];
dy = [-1, -1, -1, 0, 0, 1, 1, 1, -2, -2, -2, -1, -1, 1, 1, 2, 2, 2, -2, -2, 2, 2, -2, -2, 2];
rows = size(obstacle, 1);
cols = size(obstacle, 2);
g_score = inf(rows, cols);
f_score = inf(rows, cols);
g_score(start(1), start(2)) = 0;
f_score(start(1), start(2)) = heuristic(start, goal);
open_list = [start, f_score(start(1), start(2))];
while ~isempty(open_list)
[~, index] = min(open_list(:, 3));
current = open_list(index, 1:2);
open_list(index, :) = [];
if isequal(current, goal)
path = reconstruct_path(current);
return;
end
for k = 1:24
neighbor = current + [dx(k), dy(k)];
if is_valid(neighbor, rows, cols) && ~obstacle(neighbor(1), neighbor(2))
tentative_g_score = g_score(current(1), current(2)) + 1;
if tentative_g_score < g_score(neighbor(1), neighbor(2))
g_score(neighbor(1), neighbor(2)) = tentative_g_score;
f_score(neighbor(1), neighbor(2)) = tentative_g_score + heuristic(neighbor, goal);
if ~ismember(neighbor, open_list, 'rows')
open_list = [open_list; neighbor, f_score(neighbor(1), neighbor(2))];
end
end
end
end
end
path = []; % 无法找到路径
function h = heuristic(point, goal)
h = abs(point(1) - goal(1)) + abs(point(2) - goal(2));
end
function valid = is_valid(point, rows, cols)
valid = point(1) >= 1 && point(1) <= rows && point(2) >= 1 && point(2) <= cols;
end
function p = reconstruct_path(current)
p = current;
while isfield(current, 'parent')
current = current.parent;
p = [current; p];
end
end
end
```
代码中使用了一个二维数组 `obstacle` 表示地图,0 表示可以通过的空地,1 表示障碍物。`start` 和 `goal` 分别是起始点和目标点的坐标。函数将返回从起始点到目标点的最短路径。
该算法中使用了 24 邻域,即每个点的邻居有 24 个方向。算法会根据起始点和目标点之间的距离和当前点到目标点之间的距离进行评估,选择最优的路径进行移动。算法会在迭代过程中不断更新每个点的最短路径和估计最短路径,并将待探索的点加入开放列表中。最后,若找到可行路径,则会通过反向遍历重构路径。
以上仅是一个简单的实现示例,实际应用时可能需要对算法进行优化和适应具体场景的调整。
阅读全文
相关推荐
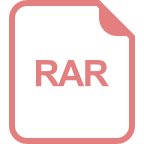
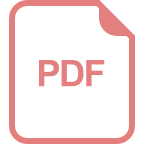
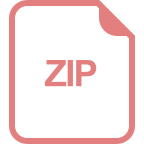













