基于stm32f103c8t6芯片的有一个蜂鸣器和ZE08甲醛传感器程序设计
时间: 2023-06-15 07:05:33 浏览: 220
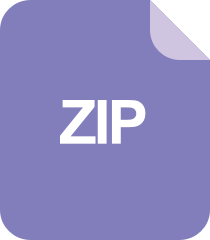
C语言开发的stm32f103c8t6实现的甲醛检测系统
以下是基于STM32F103C8T6芯片的蜂鸣器和ZE08甲醛传感器程序设计示例:
```c
#include "stm32f10x.h"
#include "stdio.h"
#define BEEP_PIN GPIO_Pin_8
#define BEEP_GPIO GPIOB
#define ZE08_TX_PIN GPIO_Pin_9
#define ZE08_RX_PIN GPIO_Pin_10
#define ZE08_GPIO GPIOA
USART_InitTypeDef USART_InitStructure;
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
/* Configure USART1 Tx (PA9) as alternate function push-pull */
GPIO_InitStructure.GPIO_Pin = ZE08_TX_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(ZE08_GPIO, &GPIO_InitStructure);
/* Configure USART1 Rx (PA10) as input floating */
GPIO_InitStructure.GPIO_Pin = ZE08_RX_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(ZE08_GPIO, &GPIO_InitStructure);
/* USART1 configuration ------------------------------------------------------*/
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
/* Enable USART1 */
USART_Cmd(USART1, ENABLE);
}
void BEEP_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = BEEP_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(BEEP_GPIO, &GPIO_InitStructure);
}
int main(void)
{
uint8_t data[9];
uint16_t value;
char str[20];
USART1_Init();
BEEP_Init();
while (1)
{
/* 读取ZE08甲醛传感器数据 */
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
if (USART_ReceiveData(USART1) == 0xFF)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
if (USART_ReceiveData(USART1) == 0x17)
{
for (int i = 0; i < 7; i++)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
data[i] = USART_ReceiveData(USART1);
}
value = ((uint16_t)data[4] << 8) + data[5];
sprintf(str, "ZE08: %d ug/m3\r\n", value);
USART_SendString(USART1, str);
/* 根据读取到的甲醛浓度值控制蜂鸣器 */
if (value > 100)
{
GPIO_SetBits(BEEP_GPIO, BEEP_PIN);
delay(100);
GPIO_ResetBits(BEEP_GPIO, BEEP_PIN);
}
}
}
}
}
```
上述代码初始化了USART1和蜂鸣器引脚,通过USART1从ZE08甲醛传感器读取数据,根据读取到的数据控制蜂鸣器。请注意,在此示例中我们使用了一个名为`delay()`的函数,它应该是用户自定义的延迟函数。您需要根据自己的需求进行相应的修改。
阅读全文
相关推荐
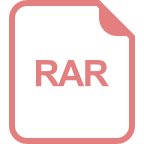
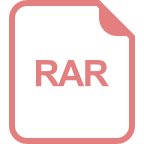

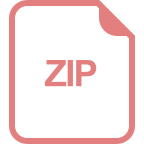
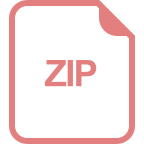
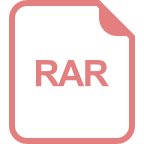
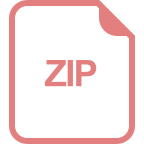
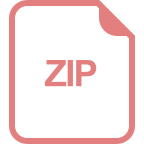
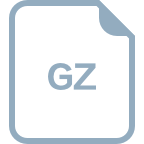
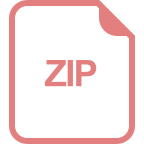
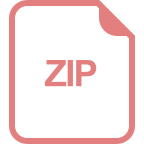
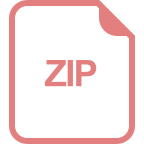
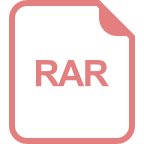
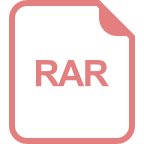
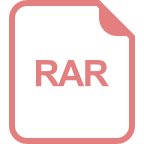