Write a Python function named "find_max" that takes a list of numbers as input and returns the maximum value from the list.use python
时间: 2024-03-10 14:45:45 浏览: 154
Sure, here is the code for the "find_max" function in Python:
```python
def find_max(numbers):
max_num = numbers[0]
for num in numbers:
if num > max_num:
max_num = num
return max_num
```
This function takes a list of numbers "numbers" as input and initializes a variable "max_num" to the first number in the list. It then loops through the rest of the numbers in the list, comparing each number to the current maximum value. If a number is found that is greater than the current maximum, it becomes the new maximum. Once all numbers have been checked, the maximum value is returned by the function.
相关问题
Write a Python function named "reverse_string" that takes a string as input and returns the reverse of the input string.
Sure! Here's a Python function named "reverse_string" that takes a string as input and returns the reverse of the input string:
```python
def reverse_string(input_str):
return input_str[::-1]
```
Here, we're using Python's string slicing notation to reverse the input string. The `[::-1]` slice means to take the entire string, but step backwards by 1 character at a time. This effectively reverses the order of the string.
Write a Python function named "is_even" that takes an integer as input and returns True if the number is even, and False otherwise.use python
Sure! Here is the code for the "is_even" function in Python:
```python
def is_even(num):
if num % 2 == 0:
return True
else:
return False
```
This function takes an integer "num" as input and uses the modulo operator (%) to check if the number is divisible by 2. If the remainder is 0, then the number is even, so the function returns True. Otherwise, the function returns False.
阅读全文
相关推荐
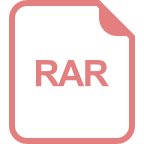
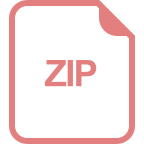
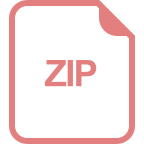






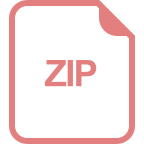
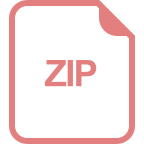
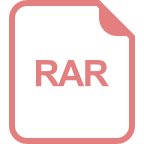
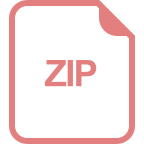
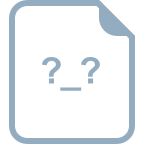
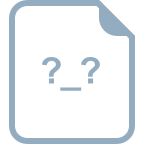
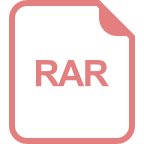