我的电脑上有2000个文件夹,每个文件夹内都有几张照片,我希望使用face++对每一张照片进行人脸检测,将每个文件夹内照片的结果整合到一个csv文件中,此csv文件中的索引为照片的文件名,最后将每个csv文件保存到对应的文件夹中。代码中包含tqdm进度条
时间: 2024-05-08 20:16:50 浏览: 88
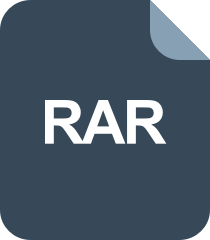
调用face++进行对图片的人脸识别
以下是Python代码实现:
```python
import os
import pandas as pd
import face_recognition
from tqdm import tqdm
# 定义人脸检测函数
def detect_faces(image_path):
image = face_recognition.load_image_file(image_path)
face_locations = face_recognition.face_locations(image)
return len(face_locations)
# 遍历所有文件夹并进行人脸检测
for foldername in tqdm(os.listdir('.')):
if os.path.isdir(foldername):
csv_file = os.path.join(foldername, 'faces.csv')
df = pd.DataFrame(columns=['filename', 'num_faces'])
for filename in os.listdir(foldername):
if not filename.endswith('.jpg') and not filename.endswith('.jpeg') and not filename.endswith('.png'):
continue
image_path = os.path.join(foldername, filename)
num_faces = detect_faces(image_path)
df = df.append({'filename': filename, 'num_faces': num_faces}, ignore_index=True)
df.set_index('filename', inplace=True)
df.to_csv(csv_file)
print('Done.')
```
解释:
1. 使用os.listdir()遍历当前目录下的所有文件和文件夹。
2. 通过判断文件夹名称是否是一个目录,来确定当前文件夹是否需要处理。
3. 对每个文件夹内的所有图片进行人脸检测,并将结果保存到一个DataFrame中。
4. 将DataFrame中的结果保存到对应的csv文件中,文件名为'faces.csv'。
5. 最后输出完成提示。
注意事项:
1. 由于face_recognition库需要安装dlib库,故在使用前需要先安装dlib库。
2. 由于人脸检测需要耗费大量的计算资源,故建议在性能较好的机器上运行本程序,或者对程序进行优化。
3. 本程序使用的是默认参数的人脸检测函数,如果需要更高的准确率或更快的速度,可以根据具体需求调整参数或使用其他函数。
阅读全文
相关推荐
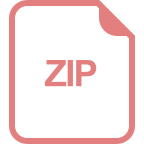
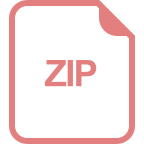
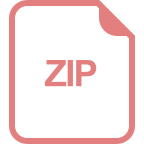
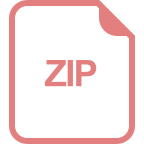
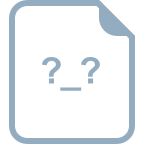
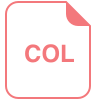
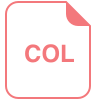
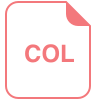
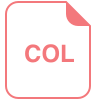



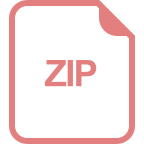
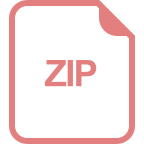
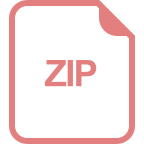