python制作英汉词典万词
时间: 2023-08-11 21:20:14 浏览: 144
要制作一个包含万词英汉词典,需要先准备好包含单词和对应中文释义的数据文件,例如CSV文件。然后使用Python读取该数据文件,并将其存储为字典数据结构。以下是一个简单的示例代码:
```python
import csv
# 读取CSV文件,将单词和中文释义存储为字典
dictionary = {}
with open('words.csv', 'r', encoding='utf-8') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
dictionary[row[0]] = row[1]
# 获取用户输入的单词并查询词典
while True:
word = input("请输入要查询的单词:")
if word in dictionary:
print("{} 的中文意思是 {}".format(word, dictionary[word]))
else:
print("抱歉,词典中没有该单词")
```
此代码使用Python内置的csv库读取CSV文件,将单词和中文释义存储为字典,接着使用循环获取用户输入的单词并查询词典。需要注意的是,此代码仅提供了基本功能,如果要实现更多功能,例如模糊匹配、单词拼写纠错等,还需要进行更多的开发和优化。
相关问题
python 英汉词典
Python英汉词典是一种基于Python语言编写的英汉词典程序。它使用了有道词典的API,可以通过输入英文单词或汉语词语来查询对应的翻译结果。具体实现过程是将用户输入的单词或词语作为参数拼接到API的URL中,然后向有道词典的服务器发送请求,得到返回的json数据,再从中提取出翻译结果并输出给用户。为了解析json数据,程序使用了simplejson模块。如果想要使用这个程序,需要先在有道词典官网上申请一个API-KEY,并将得到的API key和keyfrom以及相关参数填入程序中相应的位置。
Python英汉词典
Python英汉词典,也称为Python的词汇表或翻译工具,是一个帮助开发者查找和理解Python编程语言中英语术语及其中文意思的资源。它通常包括关键字、内置函数、模块、类库等的英文名称及其对应的中文解释。使用Python词典可以帮助非母语为英语的学习者快速掌握新概念,提高代码阅读和编写效率。
Python自带了一些官方文档,如Python官方文档(https://docs.python.org/zh-cn/),以及第三方编写的如菜鸟教程(https://www.runoob.com/python3/python3-reference.html)或一些在线词典网站(如Pydoc.org),这些都是Python英汉词典的重要来源。
阅读全文
相关推荐
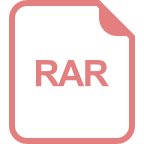
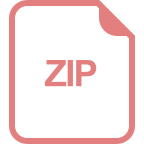
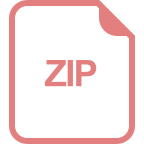
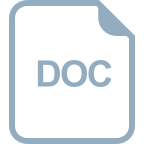
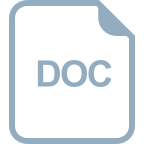




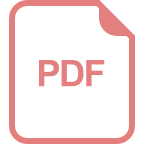