直方图均衡化、边缘增强的代码
时间: 2023-09-13 19:12:56 浏览: 72
以下是使用Python和OpenCV库实现直方图均衡化和边缘增强的代码示例:
直方图均衡化:
```python
import cv2
def histogram_equalization(image):
# Convert image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply histogram equalization
equalized = cv2.equalizeHist(gray)
return equalized
```
边缘增强:
```python
import cv2
import numpy as np
def edge_enhancement(image):
# Convert image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Gaussian blur to reduce noise
blurred = cv2.GaussianBlur(gray, (3, 3), 0)
# Apply Sobel operator to detect edges
sobelx = cv2.Sobel(blurred, cv2.CV_64F, 1, 0, ksize=3)
sobely = cv2.Sobel(blurred, cv2.CV_64F, 0, 1, ksize=3)
# Calculate gradient magnitude
gradient_magnitude = np.sqrt(sobelx**2 + sobely**2)
# Normalize gradient magnitude to 0-255 range
normalized_gradient = cv2.normalize(gradient_magnitude, None, 0, 255, cv2.NORM_MINMAX, cv2.CV_8U)
return normalized_gradient
```
你可以将以上代码复制到你的Python环境中,并传入你的图像进行测试。记得在运行代码之前,确保你已经安装了OpenCV库。
阅读全文
相关推荐






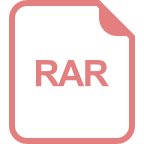


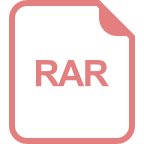








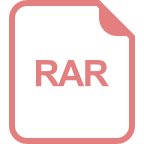