import sys import os from scapy.all import * from scapy.layers.dot11 import Dot11 # 设置网络接口为监视模式的函数 def set_monitor_mode(iface): try: comm = subprocess.Popen(['sudo','ifconfig',iface,'down'],stdout=subprocess.PIPE,stderr=subprocess.PIPE) output,error = comm.communicate() comm = subprocess.Popen(['sudo','iwconfig',iface,'mode', 'monitor'], stdout=subprocess.PIPE,stderr=subprocess.PIPE) output, error = comm.communicate() comm = subprocess.Popen(['sudo', 'ifconfig',iface,'up'], stdout=subprocess.PIPE, stderr=subprocess.PIPE) output, error = comm.communicate() except Exception as e: print("Error: {0}".format(e)) sys.exit(1) # 定义处理数据包的回调函数 def packet_callback(packet, q): # 过滤数据包中的MAC地址 if packet.haslayer(Dot11): if packet.addr2 and (packet.addr2.lower() != 'ff:ff:ff:ff:ff:ff'): # 将连接的MAC地址存入队列 q.put(packet.addr2) # 处理数据包的线程 def process_packets(q): while True: if not q.empty(): # 输出连接的MAC地址 print("Device connected: %s" % q.get()) # 主程序 if name == 'main': # 设置网络接口为监视模式 set_monitor_mode('wlan0') # 创建队列对象 q = Queue() # 创建并启动处理数据包的线程 t = Thread(target=process_packets, args=(q,)) t.daemon = True t.start() # 开始抓取数据包 sniff(prn=lambda packet: packet_callback(packet, q), iface='wlan0', filter='arp')如何在window系统下实现
时间: 2024-03-08 20:50:01 浏览: 158
这段代码需要在 Linux 操作系统下运行,因为它使用了一些 Linux 下的命令和库。在 Windows 系统下,可以考虑使用 Python 的 winpcapy 库来实现类似的功能。以下是修改后的代码:
```python
import sys
import os
from queue import Queue
from threading import Thread
from scapy.all import *
from scapy.layers.dot11 import Dot11
# 设置网络接口为监视模式的函数
def set_monitor_mode(iface):
try:
comm = subprocess.Popen(['netsh', 'interface', 'set', 'interface', iface, 'admin=disable'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
output, error = comm.communicate()
comm = subprocess.Popen(['netsh', 'interface', 'set', 'interface', iface, 'admin=enable'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
output, error = comm.communicate()
except Exception as e:
print("Error: {0}".format(e))
sys.exit(1)
# 定义处理数据包的回调函数
def packet_callback(packet, q):
# 过滤数据包中的MAC地址
if packet.haslayer(Dot11):
if packet.addr2 and (packet.addr2.lower() != 'ff:ff:ff:ff:ff:ff'):
# 将连接的MAC地址存入队列
q.put(packet.addr2)
# 处理数据包的线程
def process_packets(q):
while True:
if not q.empty():
# 输出连接的MAC地址
print("Device connected: %s" % q.get())
# 主程序
if __name__ == '__main__':
# 设置网络接口为监视模式
set_monitor_mode('Wi-Fi')
# 创建队列对象
q = Queue()
# 创建并启动处理数据包的线程
t = Thread(target=process_packets, args=(q,))
t.daemon = True
t.start()
# 开始抓取数据包
sniff(prn=lambda packet: packet_callback(packet, q), iface='Wi-Fi', filter='arp')
```
需要注意的是,这段代码需要在 Windows 操作系统下运行,并且需要使用管理员权限来执行。另外,需要安装 winpcapy 库来支持嗅探网络数据包。
阅读全文
相关推荐
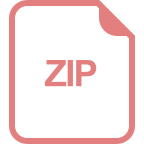
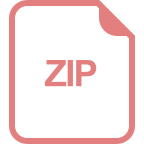
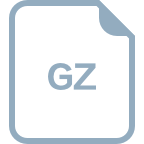
















