基于上一个问题,在项目框架为Vue3+typescript中,怎么用代码实现刚才的方案
时间: 2024-02-23 12:01:14 浏览: 145
好的,针对您的问题,我将分别介绍如何用代码实现上述方案。
1. 使用echarts提供的缩放功能。
在 Vue3+TypeScript 项目中使用 echarts,您需要先在项目中安装 echarts 和 echarts-types 类型声明文件,具体命令如下:
```
npm install echarts echarts-types -S
```
在使用 echarts 时,需要在组件中引入 echarts,然后在 created 钩子函数中初始化 echarts,具体代码如下:
```typescript
<template>
<div :style="{height: '500px'}" ref="chart"></div>
</template>
<script lang="ts">
import { defineComponent } from 'vue'
import * as echarts from 'echarts'
export default defineComponent({
setup() {
const chartRef = ref(null)
onMounted(() => {
const myChart = echarts.init(chartRef.value)
// 在这里进行图表的初始化和配置
myChart.setOption({
// echarts的配置项
...
})
})
return {
chartRef
}
}
})
</script>
```
在 echarts 中,缩放功能是通过调整图表的缩放比例实现的,可以通过以下两种方式实现缩放:
方法一:使用鼠标滚轮进行缩放
```typescript
const myChart = echarts.init(chartRef.value)
// 启用鼠标缩放
myChart.on('mousewheel', event => {
// event.event.wheelDelta 表示鼠标滚轮滚动的距离,event.event.wheelDelta > 0 表示向上滚动,event.event.wheelDelta < 0 表示向下滚动
const zoom = event.event.wheelDelta > 0 ? 1.1 : 0.9
myChart.dispatchAction({
type: 'zoom',
zoom
})
})
```
方法二:使用自定义控制器进行缩放
```typescript
const myChart = echarts.init(chartRef.value)
// 创建一个自定义控制器
const zoomController = new echarts.graphic.Rect({
left: 10,
bottom: 10,
width: 20,
height: 20,
style: {
fill: '#fff',
stroke: '#999'
}
})
// 注册控制器的鼠标事件
zoomController.on('mousedown', event => {
const mouseX = event.event.offsetX
const mouseY = event.event.offsetY
const initZoom = myChart.getOption().dataZoom[0].start
myChart.on('mousemove', event => {
const currentX = event.event.offsetX
const currentY = event.event.offsetY
const deltaY = currentY - mouseY
const zoom = Math.max(initZoom - deltaY / 100, 0)
myChart.dispatchAction({
type: 'dataZoom',
dataZoomIndex: 0,
start: zoom
})
})
myChart.on('mouseup', () => {
myChart.off('mousemove')
myChart.off('mouseup')
})
})
// 将控制器添加到图表中
myChart.getZr().add(zoomController)
```
2. 对节点进行分类展示
对节点进行分类展示,可以使用 echarts 中的系列(series)功能,具体代码如下:
```typescript
const myChart = echarts.init(chartRef.value)
myChart.setOption({
series: [
{
type: 'graph',
data: [
{
name: '节点1',
category: '类别1',
value: 10
},
{
name: '节点2',
category: '类别2',
value: 20
},
...
],
// 设置节点的样式
itemStyle: {
color: (params: any) => {
// 根据节点的类别设置不同的颜色
return params.data.category === '类别1' ? '#F00' : '#0F0'
},
...
},
// 设置节点之间的连线
edgeSymbol: ['none', 'arrow'],
...
}
]
})
```
3. 使用echarts提供的筛选功能
使用 echarts 提供的筛选功能,可以通过配置 dataZoom 组件实现,具体代码如下:
```typescript
const myChart = echarts.init(chartRef.value)
myChart.setOption({
dataZoom: [
{
type: 'slider',
show: true,
start: 0,
end: 100,
filterMode: 'filter',
xAxisIndex: 0
}
],
series: [
{
type: 'graph',
data: [
{
name: '节点1',
category: '类别1',
value: 10
},
{
name: '节点2',
category: '类别2',
value: 20
},
...
],
...
}
]
})
```
4. 对节点进行分组
对节点进行分组,可以使用 echarts 中的系列(series)功能,具体代码如下:
```typescript
const myChart = echarts.init(chartRef.value)
myChart.setOption({
series: [
{
type: 'graph',
data: [
{
name: '节点1',
category: '类别1',
value: 10
},
{
name: '节点2',
category: '类别2',
value: 20
},
...
],
// 设置节点的样式
itemStyle: {
color: (params: any) => {
// 根据节点的类别设置不同的颜色
return params.data.category === '类别1' ? '#F00' : '#0F0'
},
...
},
// 设置节点之间的连线
edgeSymbol: ['none', 'arrow'],
...
// 设置分组
categories: [
{
name: '类别1'
},
{
name: '类别2'
},
...
]
}
]
})
```
以上是用代码实现缩放、分类展示、筛选和分组的示例,您可以根据具体的业务需求进行相应的调整。
阅读全文
相关推荐
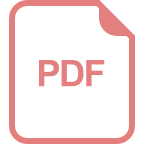
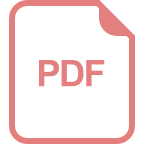
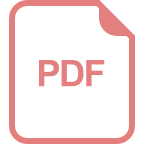
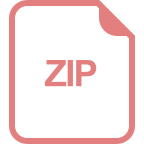
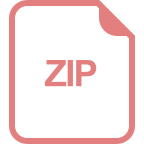
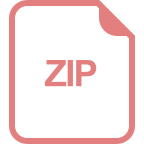
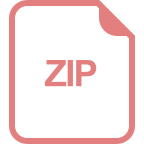
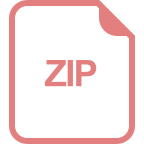
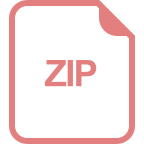
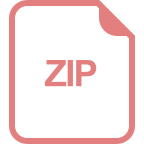
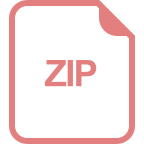
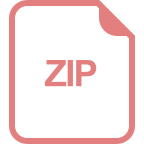
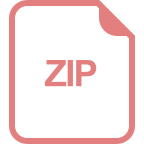
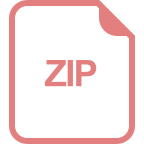
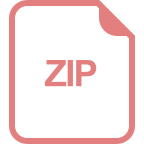
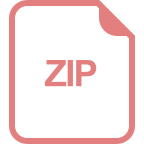
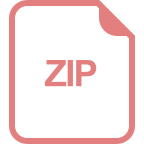
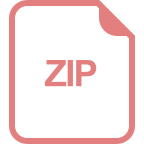