安卓蓝牙上位机开发pyqt
时间: 2023-07-04 21:12:48 浏览: 104
好的,关于安卓蓝牙上位机开发pyqt,首先需要明确的是,安卓蓝牙上位机的开发需要使用Java或Kotlin等安卓原生语言进行开发,而PyQt是Python语言的GUI库,因此在安卓上使用PyQt开发上位机并不是很常见。
但是,如果您想要在PC上使用PyQt开发蓝牙上位机程序,可以按照以下步骤进行:
1.安装PyQt库
在命令行中输入以下命令:
```python
pip install pyqt5
```
2.编写程序
根据您的需求编写程序,使用PyQt提供的控件和方法实现蓝牙上位机的功能。
3.测试程序
在PC上测试程序是否能够正常运行,并且能够与蓝牙设备进行通信。
需要注意的是,在安卓平台上使用PyQt开发蓝牙上位机需要使用第三方库进行打包和部署,具体实现方式可以参考相关文档和教程。
相关问题
pyqt5实现蓝牙上位机与stm32,代码实现
好的,以下是一个简单的示例代码,展示如何使用PyQt5实现蓝牙上位机与STM32的通信。该示例使用HC-05蓝牙模块和STM32F103开发板进行通信,使用了PyQt5中的QBluetooth和QSerialPort模块。
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QTextEdit
from PyQt5.QtCore import QIODevice, QByteArray, pyqtSignal, pyqtSlot
from PyQt5.QtSerialPort import QSerialPort, QSerialPortInfo
from PyQt5.QtBluetooth import QBluetoothDeviceDiscoveryAgent, QBluetoothSocket
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 初始化串口和蓝牙设备
self.serial_port = QSerialPort()
self.bluetooth_socket = QBluetoothSocket(QBluetoothServiceInfo.RfcommProtocol)
# 初始化界面
self.init_ui()
# 初始化蓝牙设备发现器
self.device_discovery_agent = QBluetoothDeviceDiscoveryAgent()
self.device_discovery_agent.deviceDiscovered.connect(self.device_discovered)
def init_ui(self):
# 设置主窗口标题和大小
self.setWindowTitle('Bluetooth Upper Computer')
self.setGeometry(200, 200, 500, 500)
# 设置搜索设备按钮
self.search_btn = QPushButton('Search Devices', self)
self.search_btn.setGeometry(50, 50, 100, 30)
self.search_btn.clicked.connect(self.search_devices)
# 设置连接设备按钮
self.connect_btn = QPushButton('Connect Device', self)
self.connect_btn.setGeometry(200, 50, 100, 30)
self.connect_btn.setEnabled(False)
self.connect_btn.clicked.connect(self.connect_device)
# 设置发送数据按钮
self.send_btn = QPushButton('Send Data', self)
self.send_btn.setGeometry(350, 50, 100, 30)
self.send_btn.setEnabled(False)
self.send_btn.clicked.connect(self.send_data)
# 设置文本编辑框
self.text_edit = QTextEdit(self)
self.text_edit.setGeometry(50, 100, 400, 300)
def search_devices(self):
# 开始搜索蓝牙设备
self.device_discovery_agent.start()
def device_discovered(self, device_info):
# 当发现蓝牙设备时,将其名称和地址显示在界面上
device_name = device_info.name()
device_address = device_info.address().toString()
self.text_edit.append('Device Found: ' + device_name + ' (' + device_address + ')')
def connect_device(self):
# 连接所选的蓝牙设备,并打开串口
device_address = self.selected_device.address().toString()
self.bluetooth_socket.connectToService(device_address, QBluetoothUuid.SerialPort)
self.serial_port.setPortName('COM3')
self.serial_port.setBaudRate(QSerialPort.Baud9600)
self.serial_port.setDataBits(QSerialPort.Data8)
self.serial_port.setParity(QSerialPort.NoParity)
self.serial_port.setStopBits(QSerialPort.OneStop)
self.serial_port.setFlowControl(QSerialPort.NoFlowControl)
self.serial_port.open(QIODevice.ReadWrite)
# 显示连接状态,并启用发送数据按钮
self.text_edit.append('Device Connected: ' + self.selected_device.name())
self.send_btn.setEnabled(True)
def send_data(self):
# 从文本编辑框中获取发送的数据,并发送到串口
data = self.text_edit.toPlainText().encode()
self.serial_port.write(data)
def closeEvent(self, event):
# 关闭窗口时,关闭串口和蓝牙连接
self.serial_port.close()
self.bluetooth_socket.close()
# 重载QIODevice的readyRead信号,当串口有数据可读时,将其显示在界面上
@pyqtSlot()
def ready_read(self):
data = self.serial_port.readAll()
self.text_edit.append('Received: ' + str(data, 'utf-8'))
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
以上代码仅为示例,您需要根据实际情况进行修改和完善。同时,您需要安装PyQt5、PyQt5.QtSerialPort和PyQt5.QtBluetooth模块才能正常运行该程序。希望对您有所帮助!
pyqt5开发上位机的具体流程
感谢您的提问。开发上位机的具体流程可以大致分为以下几步:
1. 确定需求:明确上位机的功能和使用场景,确定所需要的硬件设备和通讯协议。
2. 设计界面:使用pyqt5对上位机进行界面设计,包括图形界面、控件、布局等。
3. 编写代码:使用python语言编写代码实现上位机的功能,包括设备读写、数据处理和与下位机通讯等。
4. 测试调试:通过模拟实际环境或与实际设备进行通讯以及进行功能测试,修正代码中出现的问题。
5. 优化性能:对上位机的性能进行优化,提高稳定性和响应速度。
以上是开发上位机的一般流程,不同的项目有所差异。如需更具体的帮助,请提供更详细的设备和功能要求,以方便我们更好地为您解答。希望可以帮到您,谢谢。
相关推荐
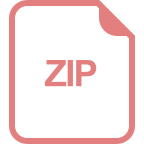
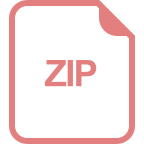












